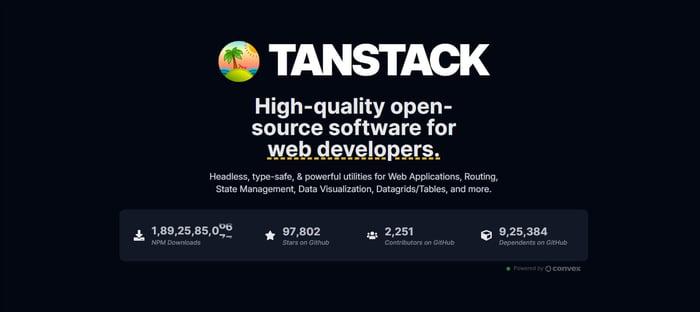
TanStack for Beginners: A Complete Guide & Tutorial
Curious about TanStack for Beginners? In this guide, we’ll explore what is TanStack, its key components, and a step-by-step TanStack tutorial. By the end, you’ll know how to use TanStack React, TanStack query, and TanStack table to build powerful apps effortlessly!
🚀 What is TanStack?
TanStack is a powerful collection of open-source libraries that simplify state management, data fetching, and table handling in modern web applications. TanStack for beginners helps developers build scalable, efficient, and high-performance applications by solving common frontend challenges like API data synchronization, dynamic table rendering, and routing.
🔍 Why Use TanStack?
TanStack tackles key pain points that developers face when dealing with complex UI states and data flow. It provides optimized solutions for:
- ⚡ Efficient Data Fetching & Caching – Eliminates unnecessary API calls, reducing load times and improving performance.
- 🧩 Seamless State Management – Handles global and local state effortlessly, avoiding prop drilling and unnecessary re-renders.
- 📊 Optimized UI Rendering – Simplifies working with large tables, making sorting, filtering, and pagination smoother.
- 🛤️ Smart Routing & Navigation – Manages dynamic routing in SPAs efficiently, with a type-safe approach.
- 🎯 Improved Developer Experience – Provides TypeScript support and intuitive APIs for easier development.
✨ Key Features of TanStack
- 📡 TanStack Query – Data Fetching & Caching
Handles API requests efficiently with automatic caching, background updates, and pagination. Ensures your app always fetches fresh data while minimizing network requests. - 📊 TanStack Table – Advanced Table Management
A powerful, lightweight, and flexible table library that supports large datasets with virtual scrolling, sorting, filtering, and pagination—without slowing down performance. - 🛤️ TanStack Router – Type-Safe Routing
A lightweight, flexible, and framework-agnostic routing solution built for React and other frameworks. It offers first-class TypeScript support, nested routes, and better state handling for SPAs. - 🎯 Performance Optimization
Uses intelligent background updates, cache invalidation, and optimized rendering to minimize unnecessary component re-renders, making applications faster and more efficient. - ⚡ TypeScript Support
Fully supports TypeScript, ensuring strong type safety, better autocompletion, and fewer runtime errors, leading to a more predictable and maintainable codebase. - 🔗 Composable & Framework-Agnostic
Works seamlessly with React, Solid, Vue, and other modern frontend frameworks, allowing developers to integrate it into their projects effortlessly.
Now that we understand what TanStack for beginners is and why it’s useful, let’s dive into how to use its core libraries—TanStack Start, TanStack Router, TanStack Query, and TanStack Table. Each of these tools solves a specific problem in modern frontend development.
🏁 Getting Started with TanStack Start (Beta)
TanStack Start is a full-stack React framework that provides built-in support for server-side rendering (SSR), streaming, API routes, and server functions. It is powered by TanStack Router, making it an ideal choice for developers who need a robust, type-safe, and optimized framework.
🔹 Why Use TanStack Start?
TanStack Start simplifies full-stack development by offering:
✅ Enterprise-grade routing – Built-in, type-safe routing using TanStack Router.
✅ SSR & Streaming – Preconfigured for full-document SSR and server-side streaming.
✅ Client-first experience – Optimized for fast-loading, interactive apps.
✅ Server Functions & APIs – Create API endpoints seamlessly.
✅ Deploy anywhere – Works with Vite and Nitro, making deployment smooth.
🚀 Setting Up TanStack Start
Let’s quickly set up a project with TanStack Start.
1️⃣ Install TanStack Start
Run the following command to create a new project:
npx create-tanstack-app@latest
You'll be prompted to choose the libraries you want to include. Select TanStack Query, Router, and Table if you want a complete setup.
2️⃣ Navigate to Your Project & Install Dependencies
After installation, go to your project directory and install dependencies:
cd my-tanstack-app
npm install
npm run dev
This will start a development server, and your app will be ready to go!
📂 Understanding the Project Structure
A TanStack Start project typically has the following structure:
my-tanstack-app/
│── src/
│ │── routes/ # Page-based routing system
│ │── components/ # Reusable components
│ │── hooks/ # Custom hooks
│ │── api/ # Server functions & APIs
│── public/ # Static assets
│── package.json
│── tsconfig.json
With this setup, routing, API handling, and state management are already configured.
🛤️ Defining Routes in TanStack Start
Since TanStack Start is powered by TanStack Router, setting up routes is straightforward.
Create a file in src/routes/index.tsx
and add:
import { createRoute, Outlet } from '@tanstack/react-router';
export const route = createRoute({
path: '/',
component: Home,
});
function Home() {
return (
<div>
<h1>Welcome to TanStack Start 🚀</h1>
<Outlet />
</div>
);
}
This creates a basic homepage with routing capabilities.
🎯 Writing Your First API Route
TanStack Start lets you create API endpoints easily. Inside the src/api/
folder, create a new file hello.ts
:
export async function GET() {
return new Response(JSON.stringify({ message: 'Hello from TanStack API!' }), {
headers: { 'Content-Type': 'application/json' },
});
}
Now, accessing /api/hello
will return:
✅ Next Steps
Now that TanStack Start is set up, you have a full-stack environment with routing and APIs ready. Next, we’ll dive into Router in TanStack for beginners, where we explore dynamic routing and navigation in detail!
🏁 Getting Started with TanStack Router
Now that we’ve set up TanStack Start, let’s explore TanStack Router, the powerful, type-safe routing solution that makes client-side navigation in React applications seamless and efficient.
🔹 Why Use TanStack Router?
TanStack Router offers:
✅ File-based & code-based routing – Supports both traditional and dynamic routing styles.
✅ First-class TypeScript support – Fully typed navigation and route definitions.
✅ Nested routing & layouts – Organize pages and components efficiently.
✅ Automatic data fetching & caching – Load data seamlessly with SWR-like caching.
✅ Built-in route loaders & prefetching – Improve performance with smarter navigation.
🚀 Installing TanStack Router
To get started, install TanStack Router in your project using your preferred package manager:
npm install @tanstack/react-router
For PNPM, Yarn, or Bun, use:
📂 Setting Up Routes
TanStack Router lets you define routes in multiple ways. The simplest way is code-based routing, where you manually define routes inside your app.
1️⃣ Create a Basic Router
In src/main.tsx
, set up the router:
2️⃣ Define Routes
Now, define routes inside src/routeTree.tsx
:
This sets up a home page and an about page, using lazy-loading for better performance.
🏗️ Using File-Based Routing
If you prefer file-based routing, use Vite's plugin to auto-generate route definitions:
npm install @tanstack/router-plugin
Then, configure it in vite.config.ts
:
Now, TanStack Router will automatically generate route definitions from your folder structure.
✅ Next Steps
At this point, you’ve set up navigation with TanStack Router! Next, we’ll explore Query in TanStack for beginners, the go-to solution for efficient data fetching and state management.
🏁 Getting Started with TanStack Query
Managing server state is challenging due to issues like caching, deduplication, background updates, and pagination.
🔹 Why Use TanStack Query?
TanStack Query simplifies server management by offering:
✅ Automatic caching & background syncing – Data stays fresh without extra API calls.
✅ Query deduplication – Prevents unnecessary network requests.
✅ Optimistic updates & mutations – Instantly update UI while waiting for the server response.
✅ Built-in pagination & infinite scrolling – Fetch large datasets efficiently.
✅ Error handling & retries – Handles API failures gracefully.
✅ Prefetching & lazy-loading – Fetch data before it's needed for a seamless UX.
To start using TanStack Query, wrap your application with QueryClientProvider
.
1️⃣ Create a Query Client
Inside src/main.tsx
, add:
2️⃣ Fetch Data Using useQuery
Now, let’s fetch some data from an API using the useQuery
hook.
Create a new component src/components/RepoData.tsx
:
This component:
✅ Fetches GitHub repo data using useQuery
.
✅ Automatically caches responses and re-fetches when data is stale.
✅ Handles loading and error states seamlessly.
Fetching data is great, but what if we need to update or send data to a server? That’s where useMutation
comes in.
3️⃣ Perform a Mutation (e.g., Adding a Todo)
Create a new component src/components/TodoList.tsx
:
This:
✅ Uses useMutation
to send data to the server.
✅ Optimistically updates the UI before waiting for confirmation.
✅ Invalidates queries to keep the UI in sync.
✅ Next Steps
At this point, you’ve set up Query in TanStack for beginners, for fetching and mutating data! Next, we’ll explore Table in TanStack for beginners,.
🏁 Getting Started with with TanStack Table
Let’s dive into TanStack Table, a headless UI library designed for building highly customizable and performance-optimized tables and data grids.
🔹 Why Use TanStack Table?
Unlike traditional table libraries that impose predefined UI elements, TanStack Table is headless, meaning it only provides the logic while leaving the styling and markup to you. It offers:
✅ Lightweight & Framework-Agnostic – Works with React, Vue, Solid, and more.
✅ Full Control Over Styling – No built-in styles, giving you complete design flexibility.
✅ Feature-Rich API – Includes sorting, filtering, pagination, row selection, and more.
✅ Optimized for Performance – Uses virtualization for handling large datasets efficiently.
🚀 Installing TanStack Table
To install TanStack Table for React, run:
npm install @tanstack/react-table
For Vue, Solid, and other frameworks, use the corresponding package:
🏗️ Building a Simple Table in React
Let’s create a fully functional table using TanStack Table.
1️⃣ Define Your Table Columns and Data
Create a new file src/components/DataTable.tsx
:
✅ Uses useReactTable
to define the table.
✅ Dynamically renders columns and rows.
✅ Provides a fully customizable structure.
Let’s enhance the table by adding sorting and search filtering.
2️⃣ Enable Sorting
Modify the column definitions to allow sorting:
Then, add sorting logic in the component:
Now, users can click column headers to sort data!
3️⃣ Adding Search Filtering
Let’s add a search bar to filter results dynamically.
Modify DataTable.tsx
:
✅ Users can search any column dynamically.
✅ Filtering works in real-time.
✅ Next Steps
You’ve now built a fully customizable table with sorting and filtering using TanStack Table! 🚀
Next, you can explore:
📌 Pagination – Load large datasets efficiently.
📌 Row selection & actions – Add interactive checkboxes.
📌 Column resizing & grouping – Optimize table layouts.
With TanStack for Beginners, you now have the power to build high-performance tables tailored to your needs!
🎯 Conclusion
In this guide, we explored TanStack for Beginners, covering its core libraries:
✅ TanStack Start – A full-stack framework for rapid development.
✅ TanStack Router – A type-safe and efficient routing solution.
✅ TanStack Query – The go-to tool for data fetching, caching, and mutations.
✅ TanStack Table – A headless UI library for building powerful, customizable tables.
By following this tutorial, you now have the knowledge to integrate TanStack libraries into your projects, improving performance, state management, and UI flexibility. Whether it's optimizing data fetching, handling navigation, or building tables, TanStack for beginners has you covered.
For more information, do check out the official Tanstack website.
Related articles
Development using CodeParrot AI
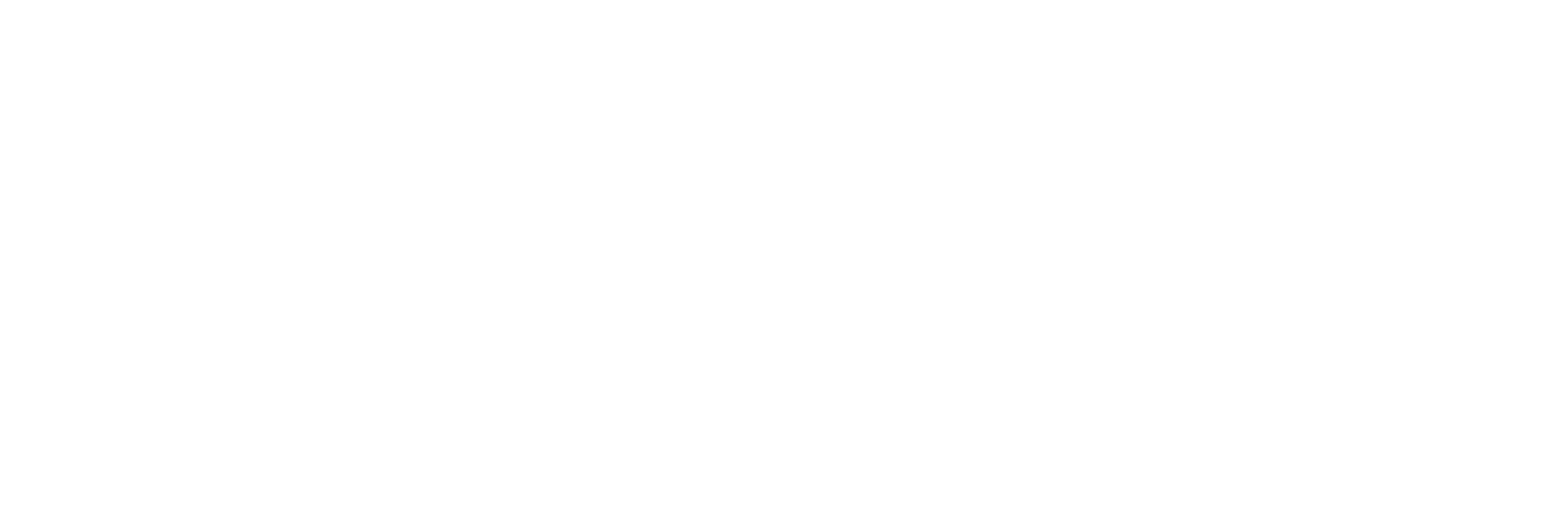