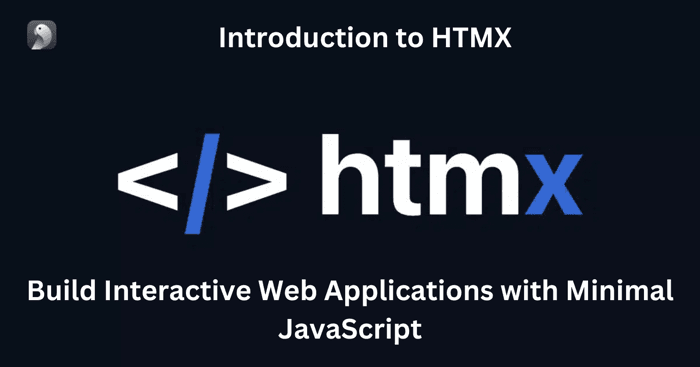
HTMX: Build Interactive Web Applications with Minimal JavaScript
Introduction to HTMX
HTMX is a lightweight JavaScript library designed to enhance web applications by enabling interactive features directly within HTML. At only 14KB (minified and gzipped), it allows developers to integrate AJAX, CSS transitions, WebSockets, and Server-Sent Events (SSE) directly from HTML attributes, bypassing the need for extensive JavaScript. This approach has made it a powerful alternative for creating interactive experiences without relying on heavy JavaScript frameworks. Unlike traditional client-side frameworks like React or Vue, which handle interactivity by managing state and logic on the client side,It shifts much of this work to the server, resulting in simpler, server-driven applications. By handling interactions through HTML attributes, makes it easy to create dynamic user interfaces without complex client-side logic.
Installation and Setup
It can be integrated into a project using several methods:
CDN: Quickly add it by including a CDN link in the HTML file’s
<head>
tag:<script src="https://unpkg.com/htmx.org@1.9.6" crossorigin="anonymous"></script>
Note: Avoid using CDNs in production for security and reliability.
Direct Download: Download
htmx.min.js
and place it in your project directory:<script src="/path/to/htmx.min.js"></script>
npm or Yarn: Install It via npm for projects using bundlers:
npm install htmx.org
Import it in your JavaScript:
import "htmx.org";
Webpack Integration: After installing It with npm, add it to Webpack:
import "htmx.org"; window.htmx = require("htmx.org"); // For global access
This setup allows bundling it with other project scripts, which is helpful for complex setups.
Core Features and Usage
AJAX Requests
It provides HTML attributes that make AJAX requests directly from HTML elements, streamlining data fetching and server communication. Here’s a summary of its main AJAX-related attributes:
hx-post
: Sends a POST request to a specified URL.hx-get
: Sends a GET request to fetch data from a URL.hx-put
: Sends a PUT request for updating resources.hx-delete
: Sends a DELETE request to remove resources.
Example:
<div hx-post="/submit">Submit Data</div>
This line of HTML will automatically send a POST request to /submit
when clicked, eliminating the need for additional JavaScript.
Triggering Events
HTMX’s hx-trigger
attribute controls when requests are triggered, allowing flexibility for different events like clicks, mouse hovers, or form submissions. This is particularly useful when you want to change the default event behavior:
mouseenter
: Initiates a request when the mouse enters the element.- Modifiers: Add extra conditions, such as
once
(trigger only once),delay:<time>
(adds delay), andthrottle:<time>
(limits frequency).
Example:
<div hx-get="/hover-data" hx-trigger="mouseenter delay:500ms">
Hover to Load Data
</div>
In this example, it will send a GET request to /hover-data
after the mouse hovers over the element for half a second.
Request Customization
It supports fine-grained control over AJAX requests through attributes like hx-params
and hx-confirm
:
hx-params
: Specifies which form fields to include in the request. For example,hx-params="*"
includes all fields, whilehx-params="field1,field2"
includes only specific fields.hx-confirm
: Adds a confirmation dialog before sending a request.
Example:
<button hx-delete="/remove-item" hx-confirm="Are you sure?">
Delete Item
</button>
This button triggers a confirmation message before executing the DELETE request, allowing users to confirm their actions before proceeding.
Advanced Usage and Interactivity
Polling and Real-Time Data
It can be used to implement polling for real-time data refresh. By setting hx-trigger
to every [interval]s
, it will automatically refresh the data at defined intervals without reloading the page. This is useful for applications where data updates frequently, like dashboards or notification panels.
Example:
<div hx-get="/notifications" hx-trigger="every 10s">
Live Notifications
</div>
This element will refresh every 10 seconds to pull the latest notifications.
Request Indicators
It provides htmx-indicator
and hx-indicator
attributes to enhance the user experience by displaying loading indicators during AJAX requests. This feedback informs users that data is being fetched.
Example:
<button hx-get="/fetch-data" hx-indicator="#loading">
Fetch Data
<span id="loading" class="htmx-indicator" style="display:none;">Loading...</span>
</button>
The htmx-indicator
class is automatically set to visible when the request is in progress and hidden afterward.
Conditional and Filtered Triggers
You can create conditional triggers with HTMX by embedding JavaScript expressions in hx-trigger
. For instance, an event can be triggered only when a specific key (e.g., ctrlKey
) is held down, making interactions more customizable.
Example:
<div hx-get="/fetch-data" hx-trigger="click[ctrlKey]">
Ctrl+Click to Fetch Data
</div>
This element will only send a request if the user holds down the control key while clicking.
DOM Manipulation and Swapping
Swapping Mechanisms
It offers multiple options for manipulating the DOM with the hx-swap
attribute, allowing developers to define how content should be inserted. Some key options include:
innerHTML
: Replaces the inner content of the target element (default).outerHTML
: Replaces the entire target element.beforeend
andafterend
: Insert content before or after the target element.
Example:
<div hx-get="/new-content" hx-swap="afterend">
Add Content After Me
</div>
Out-of-Band Swaps
HTMX’s hx-swap-oob
attribute is useful for advanced scenarios where content from a server response needs to be injected directly into the DOM, outside of the requesting element. This is particularly handy when dealing with dynamically updating sections that are not directly related to the event source.
Example:
<div id="notifications" hx-swap-oob="true">Updated Notifications</div>
<button hx-get="/get-notifications">Check Notifications</button>
In this setup, the #notifications
element will update even if it isn’t part of the requesting button’s response.
State Management with HTMX
Server-Driven State
In HTMX, state is primarily managed on the server rather than the client, contrasting with frameworks like React where state management typically happens on the client side. It's server-driven approach is beneficial for applications that don’t require extensive client-side interactions, simplifying state management and reducing the need for heavy client-side libraries.
Example:
<div hx-get="/get-data" hx-trigger="click" hx-swap="outerHTML">
Click to Get Data
</div>
Here, the server maintains the state, and It updates the DOM based on the server’s response, keeping the interface in sync with minimal client-side code.
Form Handling and Validation
It simplifies form handling by offloading submission and validation to the server. Forms can be set up to submit asynchronously, reducing client-side code complexity and ensuring that validation occurs server-side.
Example:
<form hx-post="/submit-form" hx-swap="outerHTML">
<input name="username" type="text" required />
<button type="submit">Submit</button>
</form>
In this form, the submission and any necessary validation happen on the server, making it easy to handle errors without additional client-side code.
Persistence and Complex State
For applications requiring persistence or more complex state, It can rely on server-side session state or database-driven storage. This approach works well for managing persistent data, user sessions, and long-running operations, where the server controls the state across different user interactions.
Example:
<div hx-get="/load-session-data" hx-trigger="load">
Load Session-Specific Data
</div>
This setup loads user-specific session data on page load, with the server handling persistence and complex state requirements.
Animations and CSS Transitions
It supports CSS transitions, allowing for seamless animations without extensive JavaScript. By leveraging It's htmx-swapping
class and the View Transitions API, you can create fade-in/out effects, slide animations, or other transitions when updating content.
Practical Example: Fade-Out on Content Swap
To implement a fade-out effect, you can apply a CSS transition that animates as the content is replaced.
<style>
.fade-out.htmx-swapping {
opacity: 0;
transition: opacity 0.5s ease-out;
}
</style>
<div hx-get="/new-content" hx-swap="outerHTML" class="fade-out">
Click to Load New Content
</div>
In this example, the fade-out
class reduces the element's opacity before the content swap, resulting in a smooth fade-out animation as the new content loads.
Extensions and Customization
It includes an extension framework, allowing developers to add custom functionality. Using the hx-ext
attribute, you can incorporate extensions to enhance It’s core capabilities.
Adding Extensions
To use an extension:
- Include the Extension: Add the extension’s JavaScript file to your project.
- Apply
hx-ext
: Usehx-ext
to activate the extension on specific elements.
Example:
<script src="/path/to/ext/debug.js" defer></script>
<button hx-post="/submit" hx-ext="debug">
Submit with Debugging
</button>
The debug extension will now provide additional logging for requests triggered by this button. It’s built-in extensions cover various scenarios, and you can create custom extensions to match unique project needs.
Error Handling and Logging
It offers built-in error-handling events to manage errors gracefully, providing options for custom error messages, dynamic UI feedback, and handling different HTTP error codes uniquely.
Built-In Error Handling
It triggers events like htmx:responseError
when AJAX requests fail. This event can be used to display alerts or other notifications based on the error status.
Example:
document.body.addEventListener("htmx:responseError", function (evt) {
alert("An error occurred: " + evt.detail.xhr.status);
});
Custom Error Messages and UI Feedback
To improve user experience, you can intercept errors and display messages in designated areas on the page, rather than relying on default alerts.
Example:
<div id="error-message"></div>
<button hx-get="/fetch-data" hx-target="#error-message" hx-swap="outerHTML">
Load Data
</button>
<script>
document.body.addEventListener("htmx:beforeSwap", function (evt) {
if (evt.detail.xhr.status >= 400) {
evt.detail.shouldSwap = false;
document.getElementById("error-message").innerHTML = "Something went wrong.";
}
});
</script>
In this case, errors will populate the #error-message
div, creating a more user-friendly error notification.
Granular Error Control
It enables specific handling for different HTTP error codes, allowing for customized responses. For instance, you might display a custom message for 404 errors or redirect users on a 403 error.
Example:
document.body.addEventListener("htmx:beforeSwap", function (evt) {
if (evt.detail.xhr.status === 404) {
alert("Page not found.");
evt.detail.shouldSwap = false;
} else if (evt.detail.xhr.status === 403) {
window.location.href = "/login";
}
});
This approach allows for clear, targeted error handling across various scenarios, enhancing the overall user experience by offering tailored feedback.
WebSockets and SSE with HTMX
It supports real-time data updates through WebSockets and Server-Sent Events (SSE), allowing dynamic content without frequent page reloads.
Setting Up WebSocket Connections
To establish a WebSocket connection, use the hx-ws
attribute with it, which allows you to set up real-time communication channels. This is particularly useful for live notifications, chats, and other interactive applications.
Example:
<div hx-ws="connect:wss://example.com/chat-room">
<div id="chat-messages"></div>
<form hx-ws="send:submit">
<input name="message" type="text" />
</form>
</div>
In this example, hx-ws
connects to a WebSocket, where form submissions send messages, and received messages update in real time.
Server-Sent Events (SSE)
It also supports SSE, which is ideal for one-way updates from the server, like displaying real-time updates in a feed. With hx-trigger
, It can listen for specific SSE events.
Example:
<body hx-sse="connect:/events">
<div hx-trigger="sse:new_update" hx-get="/update-feed">
Latest Updates
</div>
</body>
Here, the hx-sse
attribute connects to the server’s event stream, while hx-trigger="sse:new_update"
listens for the new_update
event and fetches the latest updates.
History and Navigation Support
It can manage browser history seamlessly with the hx-push-url
attribute. This feature helps retain URLs in the browser’s history, enabling smooth navigation and making the back/forward buttons functional within dynamic applications.
Using hx-push-url
for History Management
With hx-push-url="true"
, It automatically saves the current state and URL after each request, ensuring users can navigate back and forth smoothly.
Example:
<a hx-get="/profile" hx-push-url="true">View Profile</a>
When users click this link, the profile content loads dynamically, and the URL is saved to the history.
Tips for Enhanced Navigation
To ensure a smooth user experience:
- Use
hx-push-url
on primary links or buttons where users expect to navigate. - For fallback functionality, configure it to refresh the page if a history entry is missing, using
htmx.config.refreshOnHistoryMiss = true
.
Conclusion
HTMX offers a powerful, server-driven approach to creating interactive web applications with minimal JavaScript. Its features like AJAX, WebSockets, SSE, and CSS transitions allow for dynamic content updates directly within HTML, making it a suitable choice for developers who want to build responsive and interactive applications without heavy front-end frameworks.
In essence, It's simplicity and flexibility make it a valuable tool for building server-centric applications, delivering a seamless user experience by leveraging the power of HTML attributes for interactivity.
Related articles
Development using CodeParrot AI
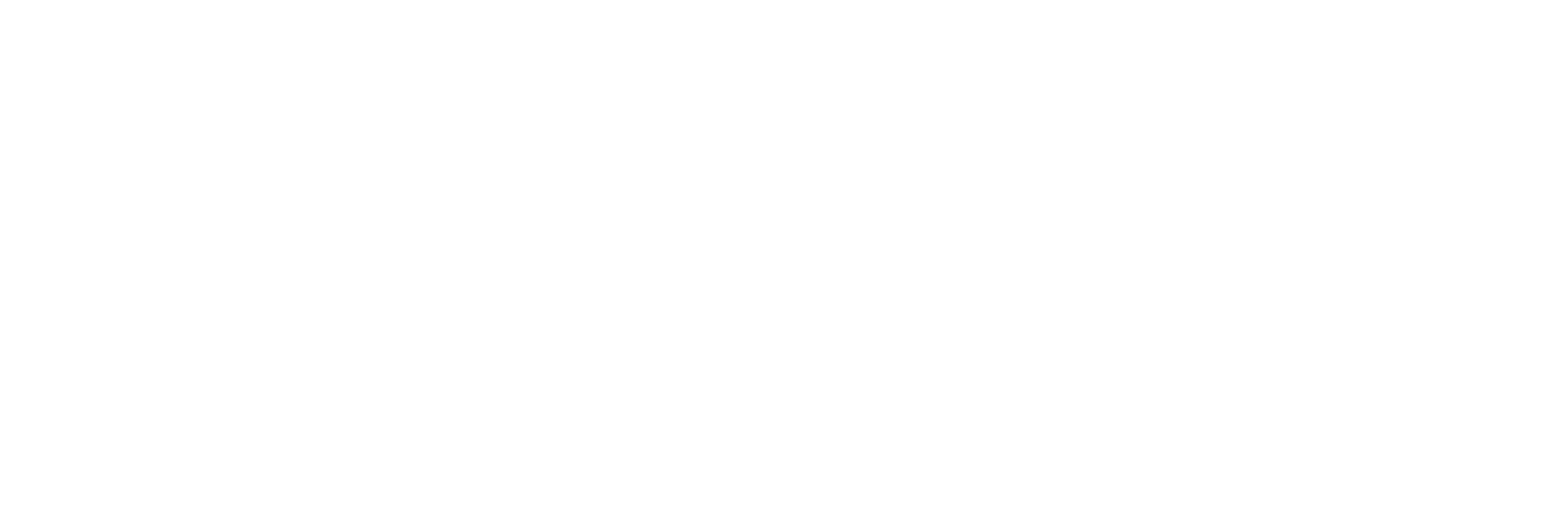