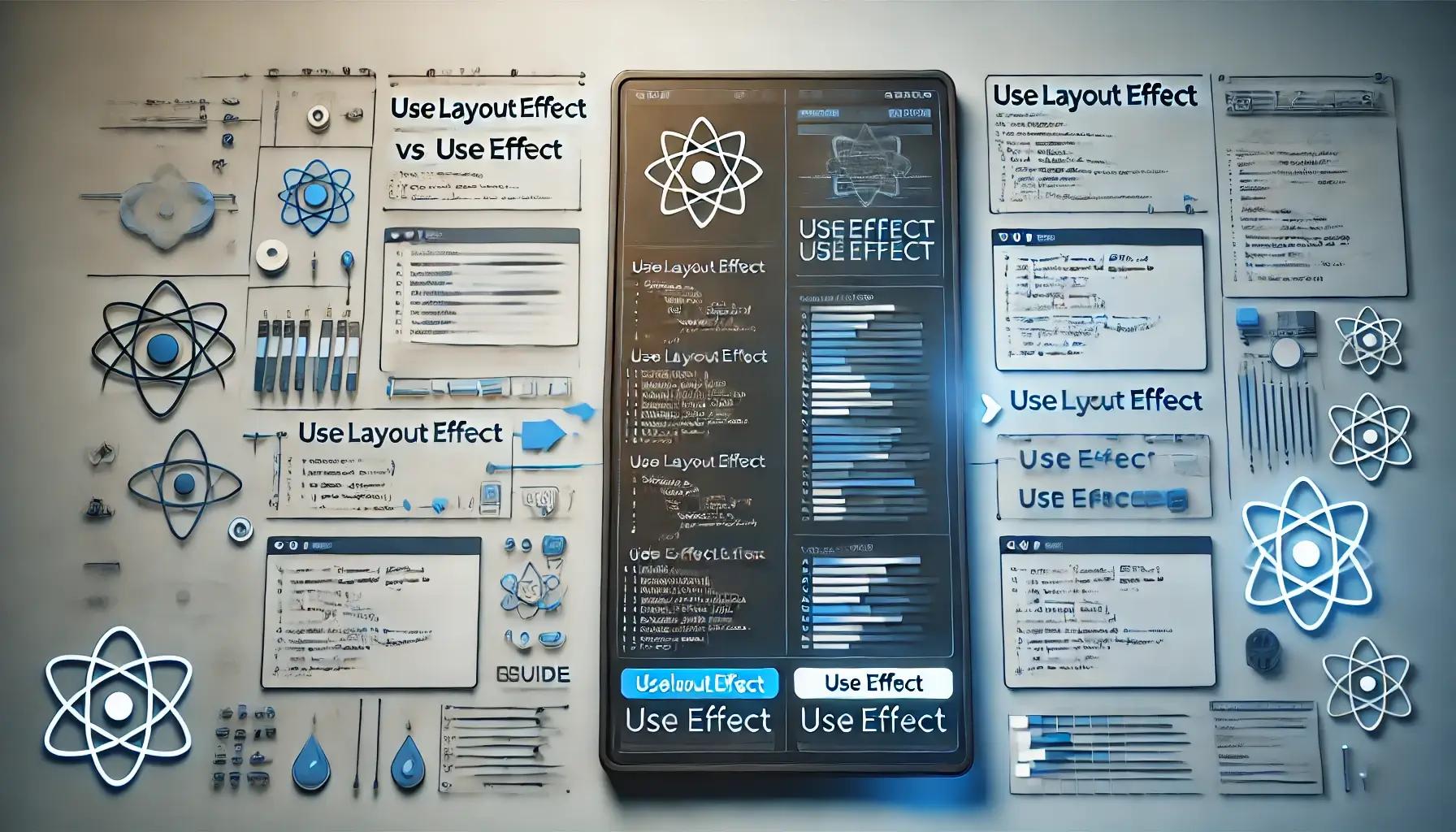
useLayoutEffect vs useEffect: A Practical Guide to React Side Effects
Introduction
React Hooks have transformed how we manage state and side effects in functional components, providing a more intuitive and flexible way to handle component logic. Among the available hooks, uselayouteffect
vs useeffect
play critical roles in managing side effects, particularly those involving DOM updates or asynchronous tasks.
Choosing the right hook is crucial for maintaining optimal performance and a smooth user experience. Both useEffect
and useLayoutEffect
can be used for similar tasks, but each has specific advantages based on execution timing and behavior. Understanding these differences helps avoid unnecessary re-renders and ensures the best possible user experience.
Understanding useEffect
Purpose
useEffect
is the go-to hook for handling side effects in React's functional components. It replaces the lifecycle methods of class components, such as componentDidMount
, componentDidUpdate
, and componentWillUnmount
, consolidating them into a single, efficient hook.
How It Works
Unlike lifecycle methods in class components that run synchronously, useEffect
executes after the component renders. This delayed execution allows the browser to update the screen before running any effects, making useEffect
non-blocking. As a result, it’s ideal for actions that don’t require immediate DOM updates, such as data fetching or event listeners.
Common Use Cases
useEffect
is versatile and widely used for tasks that involve non-blocking side effects. Here are some common scenarios where useEffect
is ideal:
Fetching data: Use
useEffect
to fetch data from an API and update the component state without affecting the initial render.useEffect(() => { async function fetchData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); setData(data); } fetchData(); }, []);
Setting up event listeners: Use
useEffect
to set up event listeners on component mount and clean up on unmount.useEffect(() => { const handleResize = () => setWindowSize(window.innerWidth); window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []);
Managing asynchronous tasks: Use
useEffect
for timers or to interact with local storage, ensuring that these tasks run after the component mounts.useEffect(() => { const timer = setTimeout(() => { setIsVisible(true); }, 1000); return () => clearTimeout(timer); }, []);
useEffect
is typically the default choice due to its non-blocking nature, making it a highly efficient way to handle most side effects without interfering with the initial render.
How useLayoutEffect
Differs from useEffect
Purpose
The primary difference between uselayouteffect vs useeffect
lies in timing and execution. While useEffect
runs after the component renders, useLayoutEffect
is specifically designed for situations where DOM manipulations need to occur immediately after rendering but before the browser paints. This timing is crucial for tasks such as measuring or adjusting DOM elements, where even a slight delay could cause visible layout shifts or flickers, disrupting the user experience.
Synchronous Execution
Unlike useEffect
, which is asynchronous, useLayoutEffect
executes synchronously. It waits until all DOM updates within it are completed, blocking the paint process until everything is applied. This synchronous behavior makes useLayoutEffect
ideal for tasks that require precise control over the DOM’s layout and appearance, helping avoid any visual inconsistencies or flickers. This distinction between uselayouteffect vs useeffect
becomes essential in situations where DOM measurements are needed for layout stability.
Example: Using useLayoutEffect
for DOM Measurements
In the example below, useLayoutEffect
is used to measure an element’s width immediately after it renders. This measurement allows for a layout adjustment before the browser paints, preventing any visible shifts.
import React, { useLayoutEffect, useRef, useState } from 'react';
function Box() {
const boxRef = useRef(null);
const [width, setWidth] = useState(0);
useLayoutEffect(() => {
setWidth(boxRef.current.offsetWidth);
}, []);
return (
<div
ref={boxRef}
style={{ width: `${width}px`, height: '100px', background: 'lightblue' }}
>
Measured Width: {width}px
</div>
);
}
In this code, useLayoutEffect
ensures that the width is accurately updated before the user sees the box on the screen. This prevents flickering that could result from delayed measurements, showcasing the key advantage of uselayouteffect vs useeffect
in maintaining a stable layout.
Choosing the Right Hook for Performance and UX
Performance Impact
useEffect
is generally more performant for non-visual effects because it runs after rendering, keeping the render process non-blocking. It’s best for actions like data fetching or event listeners, where DOM measurements aren’t required.
In contrast, useLayoutEffect
can slow down rendering if overused because it blocks the paint until its tasks are complete. Use it only when necessary to avoid performance bottlenecks.
Avoiding Visual Jank
Visual jank occurs when DOM updates create noticeable shifts on the screen. Use useLayoutEffect
to prevent this by synchronously applying layout updates before the browser paints. For example, if adjusting the height of an element based on content, useLayoutEffect
ensures this change is seamless:
useLayoutEffect(() => {
const height = calculateHeight();
setHeight(height);
}, [dependencies]);
- useEffect: Best for non-blocking, asynchronous tasks.
- useLayoutEffect: Reserved for synchronous DOM adjustments to prevent flicker.
Quick Summary and Best Practices for useLayouteffect vs useEffect
Summary Table
Feature | useEffect | useLayoutEffect |
---|---|---|
Timing | Runs after render completes | Runs after render but before the browser paint |
Execution | Asynchronous, non-blocking | Synchronous, blocks paint until complete |
Use Case | Ideal for data fetching, event listeners, and async tasks | Ideal for DOM measurements and immediate layout updates |
Performance | More performant, doesn’t block rendering | Can slow down rendering if overused |
Visual Impact | May cause flicker if used for DOM adjustments | Prevents visual jank by ensuring updates before paint |
Best Practices
When deciding between uselayouteffect vs useeffect
, following best practices can help you make the most of each hook and keep your application performant.
Default to
useEffect
: In most cases,useEffect
should be your default choice for handling side effects in React. It’s optimized for tasks that don’t impact the visible state of the DOM, such as data fetching, setting up event listeners, and managing subscriptions. BecauseuseEffect
runs asynchronously after rendering, it allows for non-blocking updates, which ensures smoother performance and prevents unnecessary delays in rendering.Reserve
useLayoutEffect
for Critical DOM Updates: UseuseLayoutEffect
only when precise control over the DOM is necessary, such as for layout measurements or adjustments that affect the visible state of an element. In scenarios where you need to measure or modify DOM properties immediately after rendering (e.g., determining the size of an element or synchronizing animations),useLayoutEffect
is the better choice in theuselayouteffect vs useeffect
decision. This helps prevent layout shifts or flickers that could disrupt the user experience.Avoid Overusing
useLayoutEffect
: WhileuseLayoutEffect
is powerful, its synchronous nature can introduce rendering delays if overused. Because it blocks the paint process until its tasks are complete, excessive use ofuseLayoutEffect
can slow down your app’s performance, especially on lower-powered devices. To optimize for performance, limituseLayoutEffect
to cases where immediate updates are absolutely necessary to maintain visual stability, and rely onuseEffect
for most other tasks.
When comparing uselayouteffect vs useeffect
, remember that useEffect
is ideal for asynchronous, non-blocking tasks, while useLayoutEffect
should be reserved for situations where immediate DOM updates are required to prevent any visual inconsistencies.
Conclusion
React offers useLayoutEffect
and useEffect
to manage side effects efficiently, each with specific strengths. While useEffect
handles asynchronous, non-blocking tasks, useLayoutEffect
addresses synchronous DOM-related updates to avoid flickers. By understanding when to use each, you can optimize your React app’s performance and enhance the user experience. Remember: start with useEffect
and reach for useLayoutEffect
only when your application demands it. This approach keeps your code clean, efficient, and visually seamless.
Related articles
Development using CodeParrot AI
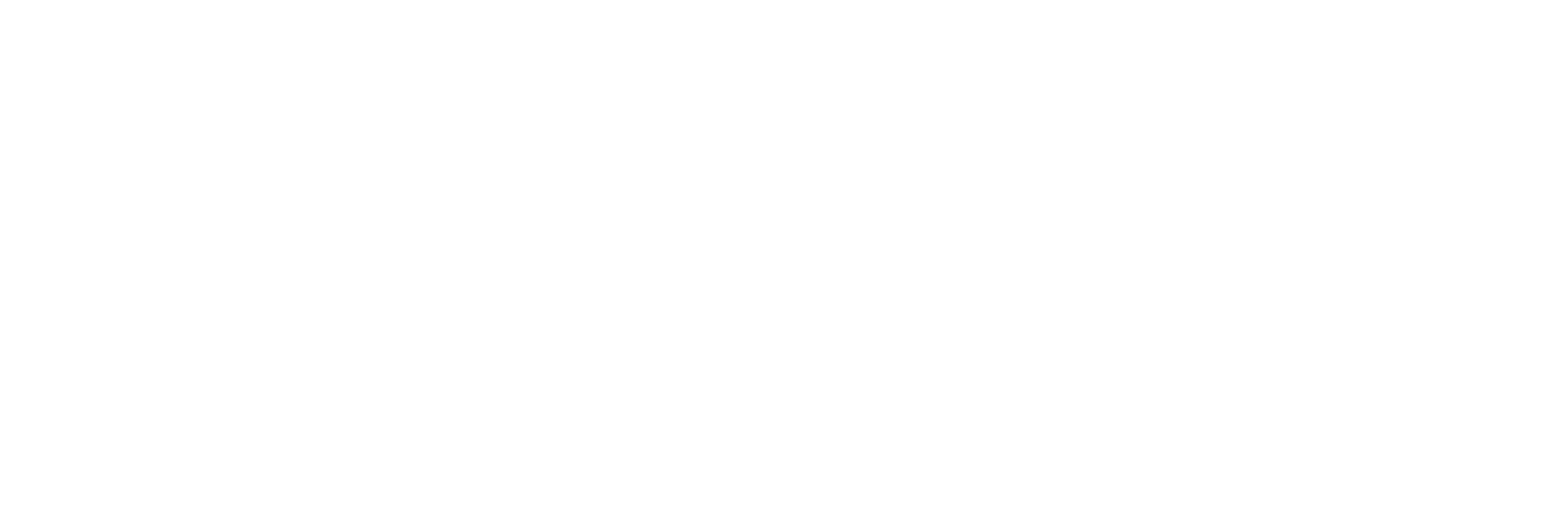