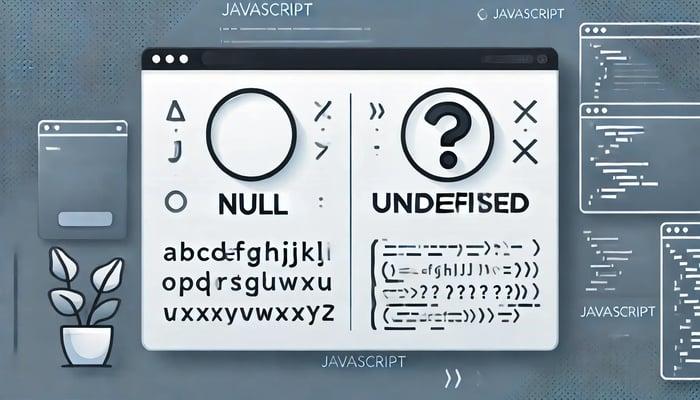
JavaScript Null vs Undefined: Key Differences & When to Use Each
In JavaScript, managing the absence of value is fundamental, and two key terms—null and undefined—serve this purpose. These two concepts play distinct roles in JavaScript’s handling of variables that lack values, each signaling a different type of "emptiness" or "absence." The comparison of null vs undefined is a central concept, especially when aiming for clarity and precision in code. By understanding these distinctions, developers can better structure their applications, avoid unexpected errors, and ensure consistency in handling variables. Let’s dive into what makes each unique.
What is null
in JavaScript?
In JavaScript, null
represents an intentional absence of value. Developers assign null
to a variable when they want to indicate that the variable exists but currently holds no meaningful data. It’s a deliberate placeholder for a value that might be assigned later or a marker to signify that the variable is empty. For example, in the case of resetting or clearing a variable's value, null
is commonly used.
let userStatus = null; // Intentionally left empty to indicate no active status yet
This usage makes null
particularly useful for cases where the absence of value is not accidental but intentional, providing a clear indicator that "no value" is a deliberate choice.
Technical Insight: The Quirk of typeof null
One of JavaScript’s long-standing quirks is that typeof null
returns "object"
. This might seem odd, as null
is clearly intended to signify an absence, not an object. This behavior originated in the early days of JavaScript and has been preserved to avoid breaking compatibility across the web. Despite this inconsistency, understanding that null
remains a primitive value helps avoid confusion:
console.log(typeof null); // Outputs: "object"
The quirky nature of null
adds a layer of complexity but does not detract from its value as a clear, intentional placeholder for "no value."
What is undefined
in JavaScript?
In JavaScript, undefined
represents a default absence of value. It signifies that a variable has been declared but has not yet been assigned any specific value. Unlike null
, which developers set intentionally, undefined
typically appears when JavaScript assigns it automatically under certain conditions.
Scenarios Where JavaScript Assigns undefined
Variable Declaration without Initialization
When a variable is declared but not initialized, JavaScript automatically assigns it the valueundefined
. This default behavior indicates that while the variable exists, it holds no meaningful data yet.let user; console.log(user); // Outputs: undefined
Accessing a Non-Existent Object Property
If you attempt to access a property that doesn’t exist on an object, JavaScript returnsundefined
. This is a way of signaling that the property is absent from the object structure.const person = { name: "Alice" }; console.log(person.age); // Outputs: undefined
Functions without a Return Statement
Functions in JavaScript that do not explicitly return a value also yieldundefined
by default. This behavior signifies that the function has completed execution without producing a specific output.function greet() { console.log("Hello!"); } console.log(greet()); // Outputs: undefined
Advanced Note: undefined
as a Global Property
undefined
is technically a property of the global object in JavaScript. Historically, this made it possible to reassign undefined
to a different value, which could lead to bugs and unexpected behavior. In modern JavaScript, while undefined
is treated more like a reserved keyword, it’s still technically possible to redefine it within local scopes. For consistency and clarity, avoid using undefined
as a variable name or identifier.
Deep Comparison Between null
and undefined
Despite their similarities, null
and undefined
have distinct purposes and behaviors. Understanding how they compare can help you make intentional choices in your code and avoid common pitfalls.
Functional Comparison: Loose Equality (==
) and Strict Equality (===
)
In JavaScript, both null
and undefined
indicate "no value," but they serve different roles. When compared using loose equality (==
), JavaScript considers null
and undefined
to be loosely equal, as they both imply an absence. However, with strict equality (===
), they are distinct because they represent different data types.
console.log(null == undefined); // Outputs: true (loose equality)
console.log(null === undefined); // Outputs: false (strict equality)
This difference highlights that while JavaScript can treat them similarly in certain comparisons, they are inherently distinct values with separate meanings.
Practical Use and Pitfalls
In some cases, null
and undefined
can appear interchangeable, but using them interchangeably can introduce bugs. The main distinction lies in their intent:
- Use
null
to represent an intentional absence—a variable intentionally set to have "no value." - Use
undefined
to represent an unintentional absence or when JavaScript’s default behavior assigns it to a variable.
Misunderstanding this distinction can lead to unintended results, especially when comparing values or when using ==
instead of ===
.
5. When to Use null
vs undefined
Choosing between null
and undefined
is essential for clear and maintainable code. Here are some guidelines to help make intentional decisions:
Use
null
when you want to mark a value as intentionally empty. This is particularly useful when you’re reserving a spot for a future value or explicitly resetting a variable. For instance, if a user logs out, you might set their session variable tonull
to indicate that it no longer holds valid information.let session = null; // Intentionally marking session as empty
Use
undefined
when you want to signify an unintentional absence of value. This is the default state for variables that are declared but not yet initialized, properties that don’t exist, or functions without return values.undefined
is generally best suited when JavaScript’s own default behavior handles the absence of value, leaving it up to the code to respond if needed.
Best Practices: Consistency in Usage
Maintaining consistent usage of null
and undefined
is especially critical in team projects. Clearly defined guidelines help prevent confusion and reduce errors. For example, teams might decide that null
should always be used as an explicit placeholder, while undefined
should represent uninitialized variables. This convention makes code more predictable and helps everyone understand the intended use of variables at a glance.
Common Pitfalls with null
and undefined
Despite their usefulness, improper handling of null
vs undefined
can lead to subtle bugs and affect code quality. Here are some common mistakes:
Reassigning
undefined
within a Scope
Whileundefined
typically represents a default absence, it is possible to reassign it within a local scope, leading to unpredictable behavior. For instance, ifundefined
is used as a variable name or redefined in a function scope, it could obscure the true meaning ofundefined
, making it harder to debug.function example() { let undefined = "some value"; // Avoid reassigning undefined console.log(undefined); // Outputs: "some value" instead of undefined }
Forgetting to Handle
null
Checks
When working with data that may containnull
values, it’s crucial to includenull
checks to avoid runtime errors. Neglecting to check fornull
in functions or when accessing object properties can lead to unexpected behavior or cause errors.let data = { user: null }; console.log(data.user.name); // Throws an error because data.user is null
Impact on Code Quality
Failing to handle null
vs undefined
properly can result in bugs that are challenging to diagnose. Additionally, inconsistent use of these values may lead to misunderstandings among developers. By clearly defining when and how to use null
and undefined
, teams can improve both the reliability and readability of their code.
Checking for null
and undefined
in Code
To avoid issues with null
and undefined
, it’s essential to use effective methods for detecting and handling them.
Practical Methods
typeof
Operator
Usingtypeof
is a quick way to check if a value isundefined
. This is especially useful in cases where a property might not exist on an object.if (typeof someVar === "undefined") { console.log("Variable is undefined"); }
Loose Equality (
== null
)
Checking== null
allows you to identify bothnull
andundefined
in a single expression. This is helpful when you want to detect any absence of value without distinguishing between the two.if (value == null) { console.log("Value is either null or undefined"); }
Strict Equality (
===
)
Use strict equality to distinguish betweennull
vsundefined
specifically. This approach is useful when you need to be explicit about the type of absence you're handling.if (value === null) { console.log("Value is null"); } else if (value === undefined) { console.log("Value is undefined"); }
Utility Functions: ??
(Nullish Coalescing)
JavaScript’s ??
(nullish coalescing) operator provides a convenient way to handle both null
and undefined
by setting a default value if either is present. It’s particularly useful for setting default values without accidentally overwriting meaningful ones like 0
or an empty string.
let name = userName ?? "Guest"; // If userName is null or undefined, name will be "Guest"
Using these techniques can help manage null
and undefined
effectively, ensuring that your code remains both resilient and readable.
Here's the conclusion with links to relevant documentation for further reference:
Conclusion: Embracing the Differences in null
vs undefined
In JavaScript, understanding the distinct roles of null
vs undefined
is essential for writing clear, robust code. While both represent an "absence of value," their uses are different by design: null
is an intentional placeholder to signal emptiness, while undefined
typically marks a default, uninitialized state. Recognizing these distinctions enables developers to communicate their intentions more clearly within the code, making it easier for others to follow and maintain.
In the ongoing comparison of null vs undefined, knowing when to use each helps prevent bugs, enhances readability, and ensures that code behaves as expected. For further reading, refer to the official JavaScript null documentation and JavaScript undefined documentation on MDN Web Docs. Mastering these concepts is a small but powerful step toward writing cleaner, more intentional JavaScript. Embracing the differences between null
vs undefined
ultimately strengthens the structure and quality of your codebase.
Related articles
Development using CodeParrot AI
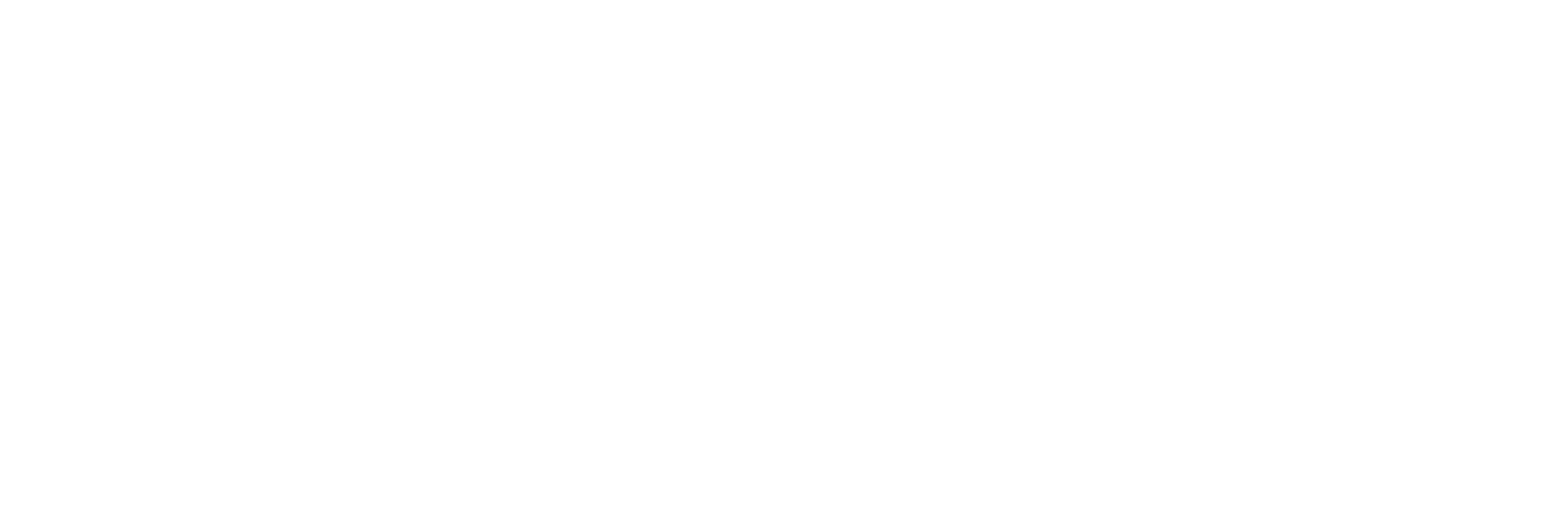