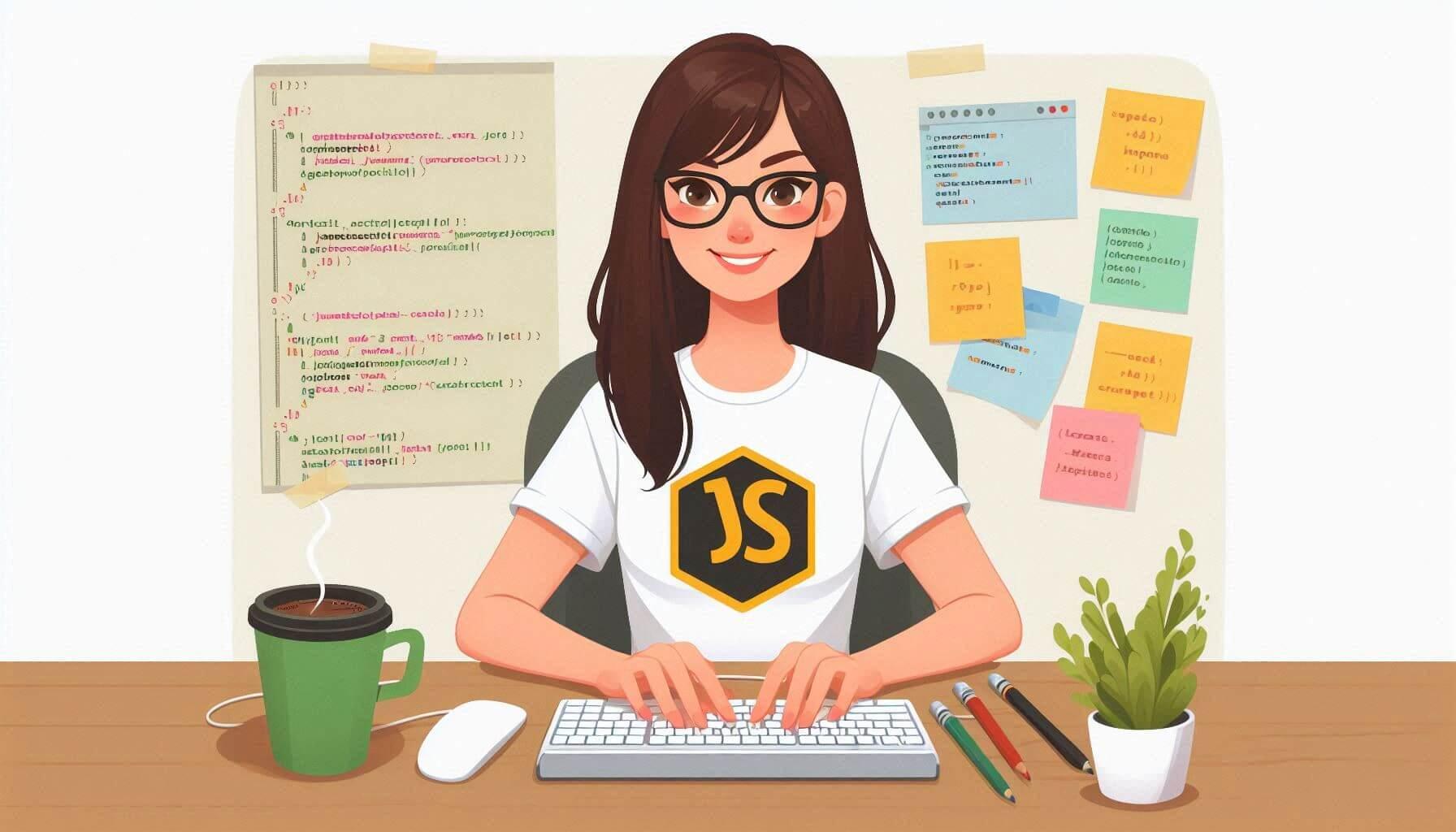
The 'this' Keyword in JavaScript: A Beginner’s Guide
In this blog, we'll dive into the "this" keyword in JavaScript, exploring how it works, why it behaves differently in various contexts, and how mastering it can make your code cleaner and more efficient. By the end, you'll have a solid grasp of how to effectively use "this" keyword in JavaScript for your projects.
So What is "this" Keyword In JavaScript?
The "this" keyword in JavaScript is essential because it enables dynamic and context-based interaction within your code. Here are some reasons why it’s so valuable:
- Access Object Properties Directly: "this" allows you to access and manipulate an object's properties and methods from within its functions, making it easier to work directly with the object.
- Event Handling: In event-driven JavaScript, "this" often refers to the HTML element that triggered the event. This makes it easier to manage DOM interactions without explicitly passing elements into functions.
- Dynamic Binding: "this" adapts based on how a function is called, not where it’s defined. This flexibility allows "this" to reference different objects, depending on context—whether it's a global function, a method inside an object, or a callback in an event listener.
- Simplifies Object-Oriented Patterns: Using "this" enables a more object-oriented approach, where properties and methods are encapsulated within objects, making code organized and scalable.
- Contextual Execution: "this" lets functions run within the context of the object that calls them. This means you don’t need to pass the object as an argument; "this" refers to it automatically.
- Helpful in Constructors and Classes: In ES6 classes or traditional constructor functions, "this" is indispensable for defining properties and methods on the newly created instance, giving each instance its own unique data.
With these features, "this" is not only a keyword but also a fundamental aspect of JavaScript’s approach to functions, objects, and context-driven coding.
Understanding the "this" Keyword in JavaScript in Different Contexts
In JavaScript, the value of the "this" keyword is not fixed and can vary depending on the context in which a function is called. This dynamic nature of "this" is one of the most unique—and sometimes confusing—aspects of JavaScript. Broadly, there are several contexts that determine the value of "this".
Let's break down each context with examples to see how "this" behaves:
1. Default/Global Context
When "this" is used in the global context or inside a standalone function, it refers to the global object, which is window
in the browser and global
in Node.js.
Example:
function showGlobalContext() {
console.log(this);
}
showGlobalContext();
This code outputs Window { ... }
in a browser or [global object]
in Node.js. Since showGlobalContext
is called in the global context, "this" points to the global object (window
in the browser or global
in Node.js). Here, there’s no explicit or implicit binding, so "this" defaults to the global scope.
2. Implicit Binding
When a function is called as a method of an object, "this" refers to the object that called the method. This is known as implicit binding.
Example:
const person = {
name: "Alice",
greet() {
console.log(`Hello, I am ${this.name}`);
}
};
person.greet();
This outputs Hello, I am Alice
because greet
is called by the person
object. Due to implicit binding, "this" inside greet
refers to person
, allowing access to its name
property. Implicit binding occurs when the function is called with a preceding object.
3. Explicit Binding
JavaScript allows for explicit binding of "this" using call
, apply
, and bind
methods. These methods let you set "this" directly to a specific object.
Example:
function introduce() {
console.log(`Hello, I am ${this.name}`);
}
const user = { name: "Bob" };
// Using call
introduce.call(user);
// Using apply
introduce.apply(user);
// Using bind
const boundIntroduce = introduce.bind(user);
boundIntroduce();
Each method invocation outputs Hello, I am Bob
. With call
and apply
, we immediately invoke introduce
, explicitly setting "this" to user
, which has a name
property of "Bob." The bind
method, however, returns a new function with "this" permanently bound to user
, allowing boundIntroduce
to be called later with "this" still set to user
.
4. Arrow Functions
Arrow functions in JavaScript don’t have their own "this" binding. Instead, they inherit "this" from their lexical scope, or the context in which they were defined. This behavior is helpful for callbacks and nested functions.
Example:
const team = {
name: "Development Team",
members: ["Alice", "Bob", "Charlie"],
introduceTeam() {
this.members.forEach(member => {
console.log(`${member} is part of ${this.name}`);
});
}
};
team.introduceTeam();
This outputs:
Alice is part of Development Team
Bob is part of Development Team
Charlie is part of Development Team
Here, the arrow function inside forEach
doesn’t create its own "this"; instead, it inherits "this" from introduceTeam
, which is called by team
. Consequently, "this" inside the arrow function refers to team
, allowing access to the name
property. If a regular function were used in forEach
, "this" would either be undefined
(in strict mode) or point to the global object, leading to unexpected results.
5. New Binding (Constructor Functions)
When a function is used as a constructor (called with the new
keyword), "this" inside that function refers to the newly created instance. This is useful for creating multiple instances of an object with their own properties and methods.
Example:
function Person(name) {
this.name = name;
}
const person1 = new Person("Alice");
console.log(person1.name); // Output: Alice
In this example, calling new Person("Alice")
creates a new object where "this" refers to that new object, not the global or any other context. The result is a new instance (person1
) with a name
property set to "Alice."
6. Class Context
In ES6+ syntax, JavaScript classes also use the "this" keyword to refer to the instance of the class in methods. The behavior is similar to new
binding, as each instance of the class will have its own "this" context.
Example:
class Car {
constructor(model) {
this.model = model;
}
showModel() {
console.log(`This car is a ${this.model}`);
}
}
const myCar = new Car("Toyota");
myCar.showModel(); // Output: This car is a Toyota
Here, "this" inside showModel
refers to the specific instance myCar
, giving access to its model
property. Each instance created with new Car
will have its own "this" referring to that instance.
7. DOM Event Listeners
In event listeners, "this" refers to the HTML element that triggered the event. This makes it easy to access the properties or methods of that element without needing to explicitly pass it as an argument.
Example:
const button = document.querySelector("button");
button.addEventListener("click", function() {
console.log(this); // Output: <button> element
});
In this case, "this" inside the event listener refers to the button element that was clicked, allowing access to its properties and methods. However, if you use an arrow function as the event handler, "this" would refer to the lexical scope, likely resulting in unexpected behavior.
Common Pitfalls with the "this" Keyword in JavaScript
Misunderstandings around "this" can lead to unexpected results in JavaScript. Here are some common pitfalls to watch out for:
1. Losing "this" in Callback Functions
When passing a method as a callback, "this" can lose its original reference. This happens because when a function is called as a standalone (without an object calling it), "this" defaults to the global object or becomes undefined
in strict mode.
Example:
const user = {
name: "Alice",
greet() {
console.log(`Hello, I am ${this.name}`);
}
};
setTimeout(user.greet, 1000); // Output: Hello, I am undefined
In this example, this
becomes undefined within greet
because setTimeout
calls greet
as a standalone function, not as a method of user
.
2. Unexpected "this" in Arrow Functions
Arrow functions don’t have their own "this" context; instead, they inherit "this" from the surrounding lexical scope. This can cause issues when arrow functions are used in situations where "this" should refer to the calling object, such as in methods or event listeners. This behavior can lead to unexpected values for "this" in scenarios where developers might expect a new "this" context.
Example:
const button = document.querySelector("button");
button.addEventListener("click", () => {
console.log(this); // Output: Window object (or global object in Node.js)
});
Here, "this" refers to the global object instead of button
because arrow functions inherit "this" from their defining scope, rather than from the event context.
3. "this" in Nested Functions
When using regular functions nested within methods, "this" may unexpectedly point to the global object rather than the outer function or object. This happens because each function invocation has its own "this" context. In a nested function, if "this" isn’t bound explicitly, it defaults back to the global context, which can lead to unexpected behavior when trying to access properties of the outer object.
Example:
const person = {
name: "Alice",
introduce() {
function showName() {
console.log(this.name);
}
showName(); // Output: undefined (or error in strict mode)
}
};
person.introduce();
In this example, "this" inside showName
defaults to the global scope rather than referring to person
, leading to an unexpected output.
Best Practices for Using the "this" Keyword in JavaScript
Mastering the "this" keyword in JavaScript can greatly improve code readability and maintainability. Here are some best practices to help ensure "this" behaves as expected in various contexts:
1. Use Arrow Functions for Lexical Scoping
For functions that need to retain "this" from the surrounding scope, use arrow functions. Arrow functions don’t have their own "this," so they inherit it from where they were defined. This is helpful in callbacks or nested functions.
Example:
const person = {
name: "Alice",
introduce() {
setTimeout(() => {
console.log(`Hello, I am ${this.name}`);
}, 1000);
}
};
person.introduce(); // Output: Hello, I am Alice
2. Use Bind, Call, or Apply for Explicit Binding
When you need to set "this" to a specific object, use bind
, call
, or apply
. This is useful for callbacks or standalone function calls where you want "this" to reference a specific object.
Example:
const user = {
name: "Bob",
greet() {
console.log(`Hello, I am ${this.name}`);
}
};
setTimeout(user.greet.bind(user), 1000); // Output: Hello, I am Bob
3. Avoid "this" in Global Scope
In the global scope, "this" refers to the window
(in browsers) or global
(in Node.js) object, which can lead to unexpected results. Keep "this"-dependent functions within objects or classes.
Example:
function showThis() {
console.log(this);
}
showThis(); // Output: window or global object
4. Use "this" in Classes and Constructors
In ES6 classes or constructor functions, use "this" for instance properties. This keeps each instance’s data separate, following object-oriented design.
Example:
class Car {
constructor(model) {
this.model = model;
}
showModel() {
console.log(`This car is a ${this.model}`);
}
}
const myCar = new Car("Toyota");
myCar.showModel(); // Output: This car is a Toyota
5. Test "this" in Various Contexts
Test how "this" behaves when your function is used in different contexts—such as methods, callbacks, and event listeners. This helps catch unexpected results early in development.
Conclusion
In this blog, we've explored the "this" keyword in JavaScript, covering its behavior in various contexts like global, implicit, explicit, new
binding, and arrow functions. We also discussed common pitfalls to avoid and best practices to ensure "this" works as expected in your code. Mastering "this" can greatly improve code clarity and flexibility, empowering you to write more efficient and maintainable JavaScript.
For more in-depth exploration, feel free to checkout the MDN documentation on "this" keyword in JavaScript.
Related articles
Development using CodeParrot AI
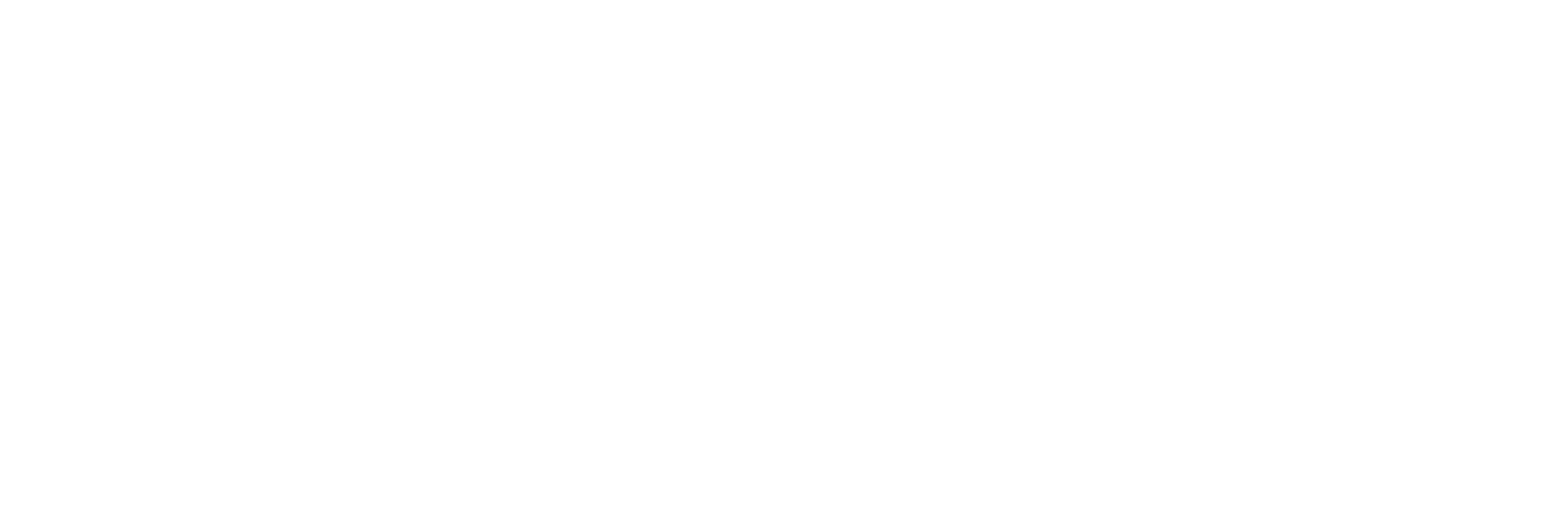