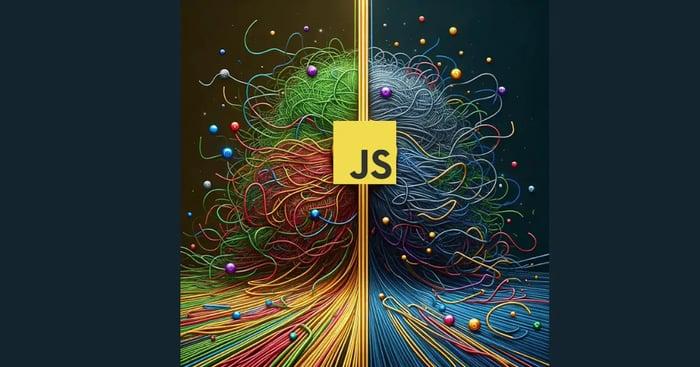
Worker Threads: Javascript is not single threaded!
What if I say you that JavaScript is not strictly single-threaded and that there's a way to harness the power of multithreading through worker threads? Intrigued? Let's dive deeper into this topic.
JavaScript has long been perceived as a single-threaded environment, primarily because of its single-threaded event loop that handles asynchronous tasks, UI rendering, and user interactions in a sequential manner. This design choice helps simplify the development process but can lead to performance bottlenecks when executing long-running tasks.
Worker threads are JavaScript's answer to achieving parallelism, allowing you to run scripts in background threads and perform heavy tasks without interrupting the main thread. This means you can execute computationally expensive operations, such as data processing or complex calculations, without compromising the responsiveness of your web application. They allow you to execute JavaScript in parallel, offering a solution for offloading processing tasks from the main event loop, thus preventing it from getting blocked.
- Parallel Execution: They run in parallel with the main thread, allowing CPU-bound tasks to be handled more efficiently.
- Non-Blocking: By delegating heavy lifting to threads, the main thread remains unblocked, ensuring smooth performance for I/O operations.
- Shared Memory: Threads can share memory using
SharedArrayBuffer
, facilitating efficient communication and data handling between threads.
Worker threads, stabilized in Node.js version 12, offer an API for managing CPU-intensive tasks effectively. Unlike clusters or child processes, it can share memory, allowing for efficient data transfer between threads. Each worker operates alongside the main thread, equipped with its event loop, enhancing the application's capability to handle multiple tasks concurrently.
A Simple Example
Consider a Node.js application where the main thread is tasked with a CPU-intensive operation, such as image resizing for different use cases within an app. Traditionally, this operation would block the main thread, affecting the application's ability to handle other requests. By offloading this task to a worker thread, the main thread remains free to process incoming requests, significantly improving the application's performance and responsiveness.
Code Snippet for Image Resizing
// main.js
const { Worker } = require("worker_threads");
function resizeImage(imagePath, sizes, outputPath) {
const worker = new Worker("./worker.js", {
workerData: { imagePath, sizes, outputPath },
});
worker.on("message", (message) => console.log(message));
worker.on("error", (error) => console.error(error));
worker.on("exit", (code) => {
if (code !== 0)
console.error(new Error(`Worker stopped with exit code ${code}`));
});
}
// worker.js
const { parentPort, workerData } = require("worker_threads");
const sharp = require("sharp"); // Assuming sharp is used for image processing
async function processImages() {
const { imagePath, sizes, outputPath } = workerData;
try {
for (const size of sizes) {
const output = `${outputPath}/resize-${size}-${Date.now()}.jpg`;
await sharp(imagePath).resize(size.width, size.height).toFile(output);
parentPort.postMessage(`Image resized to ${size.width}x${size.height}`);
}
} catch (error) {
parentPort.postMessage(`Error resizing image: ${error}`);
}
}
processImages();
In this example, the main thread initiates a worker thread for resizing an image, passing the necessary data through workerData
. The worker thread performs the resizing operation using the sharp
library and communicates the progress back to the main thread without blocking it.
Worker threads are not a panacea for all performance issues but are particularly effective in scenarios involving CPU-intensive operations such as:
Machine Learning in JavaScript
With the rise of JavaScript-based machine learning libraries such as TensorFlow.js, there's an increasing demand for handling intensive computations directly within JavaScript applications. It can be leveraged to run machine learning models, perform training, and process large datasets in the background.
Real-Time Data Processing
In applications that require real-time data processing, such as financial trading platforms or IoT systems, threads can process incoming data streams concurrently. For instance, a Node.js server receiving a continuous stream of sensor data can use threads to parse, analyze, and store data without delaying the main event loop. This ensures that the system remains responsive and capable of handling high-throughput data.
Game Development
JavaScript is increasingly used in game development, particularly with frameworks like Phaser.js. Games often require real-time rendering, physics calculations, and AI processing, which can be CPU-intensive. Offloading these tasks to threads can significantly enhance performance, providing a smoother gaming experience. By parallelizing tasks such as pathfinding algorithms or complex collision detection, threads can help maintain a consistent frame rate and improve overall gameplay.
- Security: Keep in mind that workers have some limitations for security reasons, such as no access to the DOM.
- Performance: While workers are powerful, spawning too many workers can lead to memory and performance issues. Always test and optimize.
Challenges and Limitations
Memory Overhead
Each worker thread runs its own instance of the V8 engine, leading to increased memory usage. While this allows for true parallel execution, it can also introduce overhead, especially if many worker threads are spawned simultaneously. Developers must balance the number of worker threads with available system resources, potentially implementing a pool of reusable worker threads to manage resource utilization effectively.
Thread Management
Efficient management of worker threads is essential to prevent resource exhaustion. Implementing a worker pool can help manage the lifecycle of threads, reusing them for different tasks rather than creating and destroying threads frequently. This approach reduces the overhead associated with thread creation and teardown, leading to better performance and resource management.
Communication Overhead
While worker threads can share memory using SharedArrayBuffer, developers must be cautious about the overhead associated with inter-thread communication. Passing large amounts of data between threads can be costly in terms of performance. Optimizing the data transfer mechanisms and minimizing the amount of data shared between threads can help mitigate this issue.
Worker threads represent a significant advancement in JavaScript's capabilities, allowing developers to harness the power of multithreading for improved performance and responsiveness. By understanding the benefits, use cases, and challenges associated with worker threads, developers can make informed decisions about when and how to implement them in their projects. Whether you're building complex machine learning applications, real-time data processing systems, or interactive games, worker threads can help you achieve a higher level of performance and scalability.
Related articles
Development using CodeParrot AI
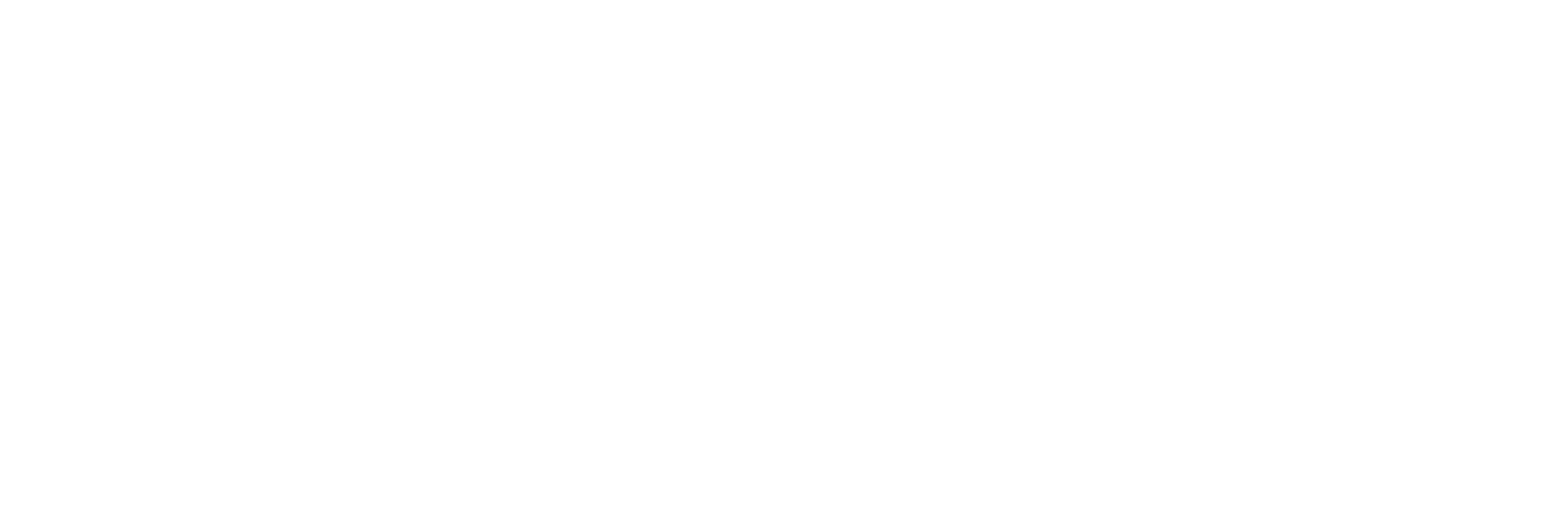