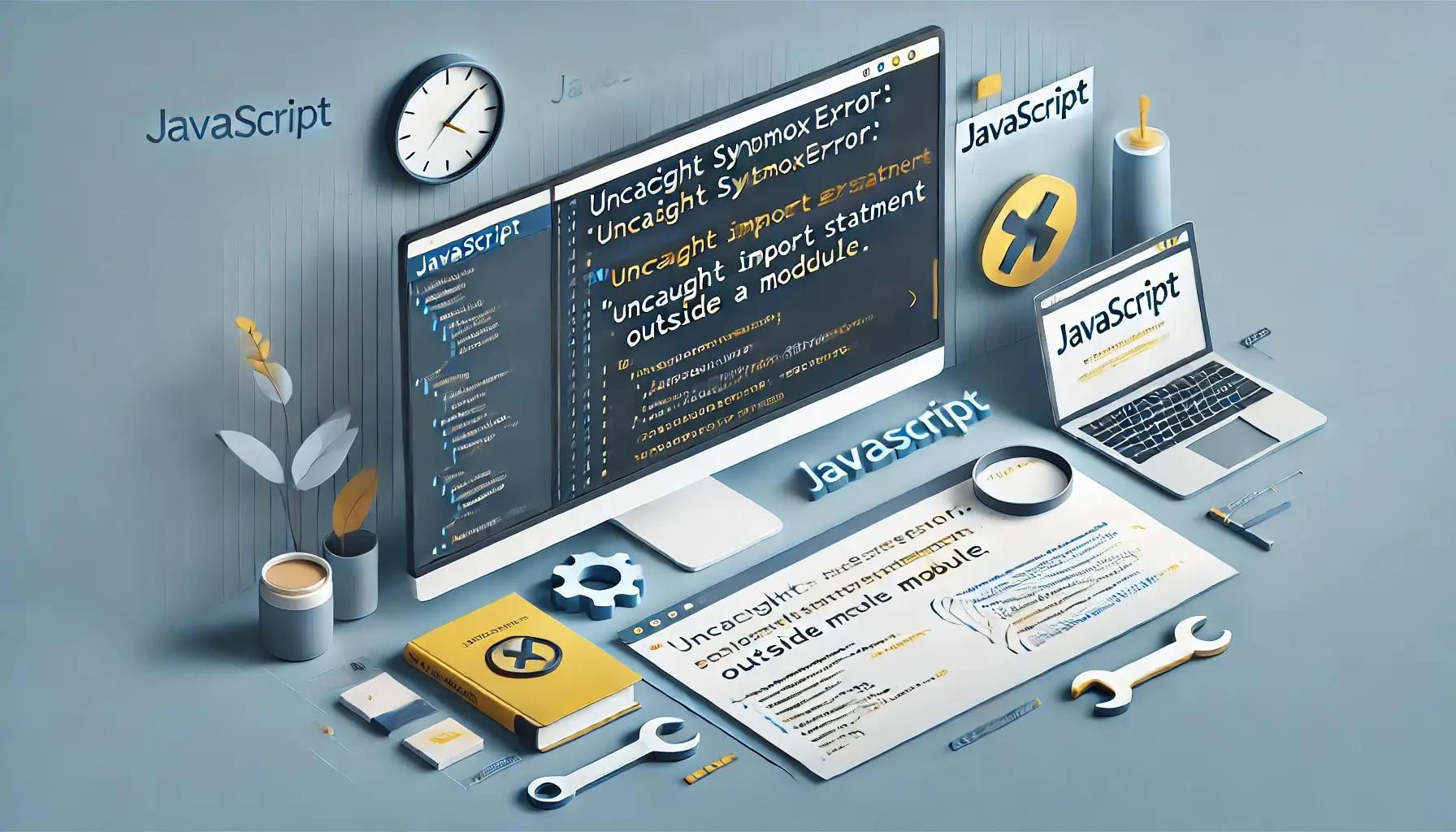
Understanding and Fixing 'Uncaught SyntaxError: Cannot Use Import Statement Outside a Module' in JavaScript
Modern JavaScript empowers developers to write cleaner, modular code using ECMAScript Modules (ES Modules). These modules simplify code organization by enabling the use of import
and export
statements, making it easier to manage dependencies and maintain scalability in larger applications. However, transitioning to ES Modules isn’t always seamless. Developers often encounter the following error: Uncaught SyntaxError: Cannot use import statement outside a module
This error, officially referred to as the "syntaxerror: cannot use import statement outside a module," typically occurs when a JavaScript file is not properly recognized as a module, even though it uses ES6 syntax. While the error message is straightforward, the root cause often involves misconfigurations or misunderstandings about how JavaScript modules work.
This guide aims to demystify this error by exploring its causes and providing practical solutions for both browser and Node.js environments. By the end, you’ll have the knowledge needed to fully leverage ES Modules in your JavaScript projects.
The Problem
The error Uncaught SyntaxError: Cannot use import statement outside a module
often arises when attempting to use ES6 module syntax (import
and export
) in an environment that isn’t properly set up to recognize it. For example:
// main.js
import { greet } from './helper.js';
greet();
And the helper.js
file contains:
// helper.js
export function greet() {
console.log("Hello, world!");
}
When running the above code in a browser or Node.js environment that does not treat helper.js
as a module, you’ll encounter the "syntaxerror: cannot use import statement outside a module" error. The issue lies in how JavaScript determines whether a file should be treated as an ES Module.
This problem becomes especially frustrating for developers transitioning from older JavaScript practices or working with mixed environments (e.g., legacy systems using CommonJS).
Why Does This Happen?
The "syntaxerror: cannot use import statement outside a module" error stems from differences in how JavaScript environments interpret files and their configurations.
Browser Environments
Modern browsers support ES Modules natively, but you must explicitly declare your script as a module. This is done by adding the type="module"
attribute to the <script>
tag. Without this declaration, the browser treats the file as a regular script, which does not support import
and export
statements.
For example:
<!-- Without type="module" -->
<script src="main.js"></script>
<!-- Results in the error -->
<!-- With type="module" -->
<script type="module" src="main.js"></script>
Modules in Browsers
- Deferred Execution: ES Modules are deferred by default, meaning they don’t block the HTML parsing process.
- Strict Mode: All ES Modules automatically run in strict mode, helping catch common errors.
Node.js Environments
Node.js traditionally used CommonJS as its module system, which relies on require
and module.exports
. While ES Modules are supported starting from Node.js version 12.x
, they require explicit configuration. If this configuration is missing, Node.js will default to treating .js
files as CommonJS, causing the error.
What Are ES Modules?
ES Modules, introduced in ECMAScript 6 (ES6), provide a standardized way to manage dependencies in JavaScript. They allow developers to:
- Export specific parts of a file, such as functions, variables, or classes, using the
export
keyword. - Import these parts into other files using the
import
keyword.
This system promotes encapsulation and reduces issues caused by global variables. For example:
// helper.js
export function greet() {
console.log("Hello, world!");
}
// main.js
import { greet } from './helper.js';
greet();
With ES Modules, sharing functionality between files becomes explicit and maintainable, especially in large projects. Modules are also an essential component of modern build tools like Webpack, Vite, and Rollup.
CommonJS vs. ES Modules
Before ES Modules, Node.js used CommonJS, which is still widely supported for backward compatibility. Here’s a comparison:
Feature | CommonJS | ES Modules |
---|---|---|
Syntax | require and module.exports | import and export |
Runtime Loading | Synchronous | Asynchronous |
Standardization | Node.js-specific | ECMAScript standard |
Node.js Support
- Versions prior to 12.x use CommonJS exclusively.
- From version 12.x onwards, ES Modules are supported but require configuration (e.g., using
.mjs
files or adding"type": "module"
topackage.json
).
Browser Support
Modern browsers natively support ES Modules. However, the type="module"
attribute must be added to <script>
tags for the browser to recognize the file as a module.
Transition Challenges
One key challenge for developers is working with both CommonJS and ES Modules in the same project. While tools like Babel and Webpack help bridge the gap, understanding the differences and when to use each system is crucial.
The Solution
For Browser Environments
To ensure the browser recognizes your JavaScript file as an ES Module, include the type="module"
attribute in your <script>
tag:
<!DOCTYPE html>
<html lang="en">
<head>
<title>ES Modules Example</title>
</head>
<body>
<script type="module" src="./main.js"></script>
</body>
</html>
Key Benefits
- Organized Code: Break your application into reusable modules.
- Native Support: Modern browsers like Chrome, Firefox, and Safari handle ES Modules without additional tools.
For Node.js Environments
Option 1: Update package.json
Add "type": "module"
to your package.json
file:
{
"name": "es-modules-example",
"type": "module"
}
This tells Node.js to treat all .js
files in the project as ES Modules.
Option 2: Use the .mjs
Extension
Rename your JavaScript files to have the .mjs
extension, which explicitly marks them as ES Modules:
mv main.js main.mjs
mv helper.js helper.mjs
node main.mjs
Option 3: Use an ESM Loader for Older Versions
For Node.js versions without native ES Module support, use the esm
loader:
npm install esm
node -r esm main.js
Advanced Tips and Best Practices
Combining CommonJS and ES Modules
If mixing modules is unavoidable, use dynamic imports to load CommonJS modules in ES Modules:
const { readFile } = await import('fs');
Dynamic imports enable conditional and asynchronous loading, which is useful in complex projects.
Default vs. Named Exports
Default Exports: Use when exporting a single main function or class:
export default function greet() { console.log("Hello!"); }
Named Exports: Use when exporting multiple items:
export const name = "JavaScript"; export function version() { return "ES6"; }
Cross-Browser Compatibility
For older browsers:
- Use Babel to transpile ES Module syntax into compatible JavaScript.
- Use Webpack to bundle modules for consistent delivery.
Conclusion
ES Modules offer a powerful and standardized framework for managing dependencies, improving code organization, and promoting maintainability. However, their adoption requires attention to configuration and compatibility.
By understanding the causes of errors like Uncaught SyntaxError: Cannot use import statement outside a module
and following the solutions outlined here, you can avoid common pitfalls and fully leverage the benefits of ES Modules. Embracing best practices—such as sticking to a single module system and ensuring cross-environment compatibility—will enable you to build scalable, future-proof applications.
For more insights on JavaScript module systems, check out our related articles:
- CommonJS vs. ES Modules: Key Differences Explained
- How to Use Babel for Cross-Browser JavaScript Compatibility
- Mastering JavaScript Imports and Exports for Clean Code
These resources can further enhance your understanding of modular JavaScript development. Happy coding!
Related articles
Development using CodeParrot AI
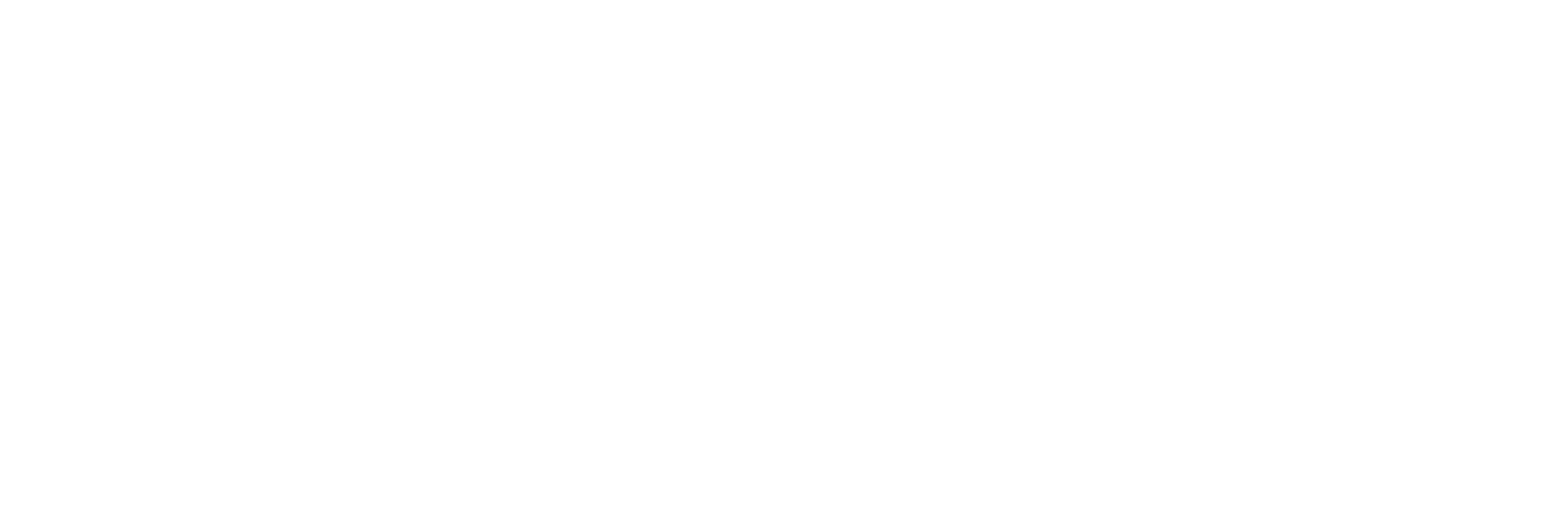