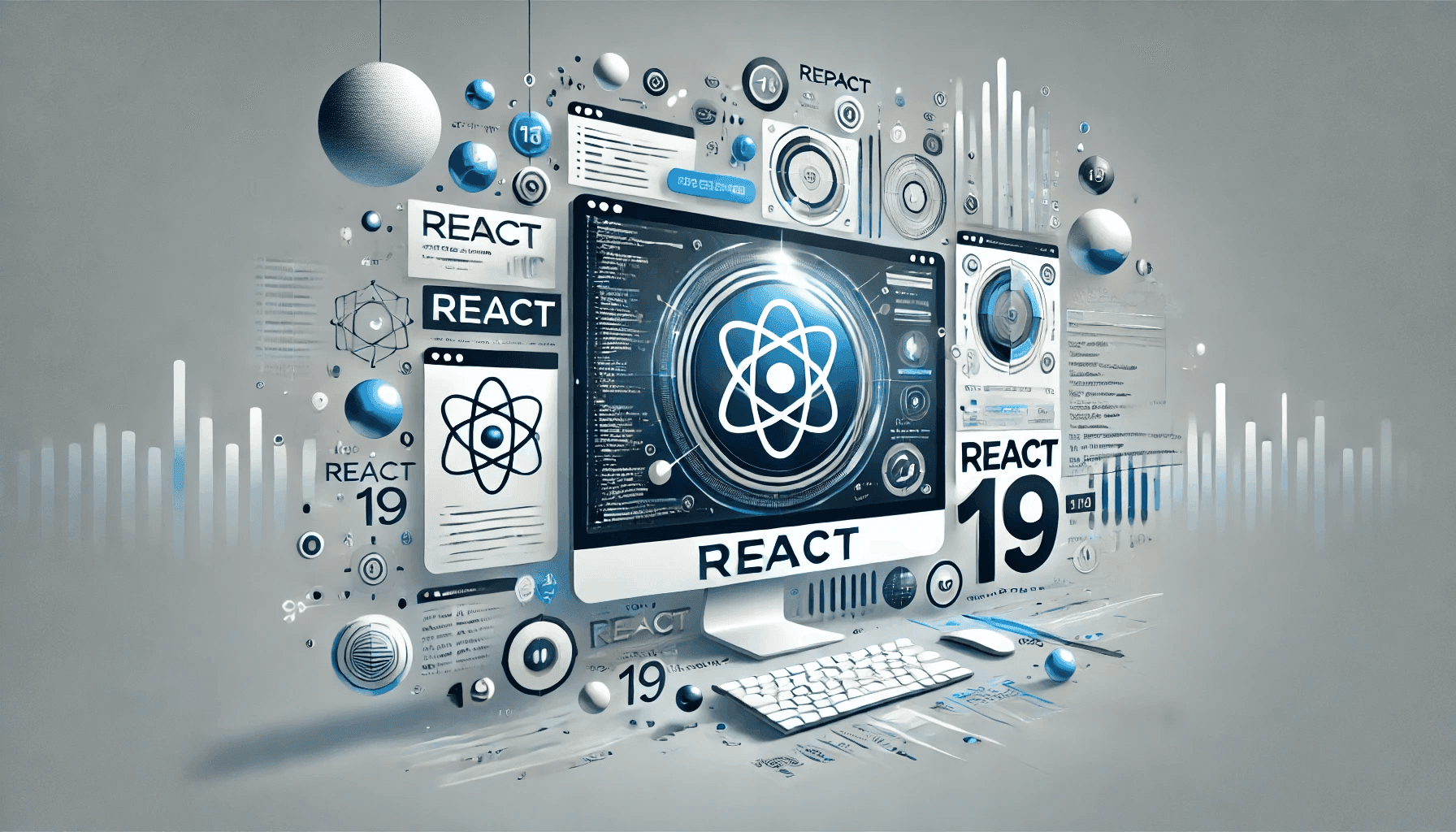
React 19: A Comprehensive Guide
React 19 is here! 🎉 In this article, we'll explore the new features and improvements in React 19. Let's dive in!
What's New in React 19
React 19, the latest major release from the React team, comes with several groundbreaking features and enhancements designed to make the development process more efficient and the resulting applications faster and more powerful. This release continues to build on the strong foundation laid by its predecessors, introducing significant updates to the core framework. In this blog, we’ll explore the key features of React 19, including the new React Compiler, Server Components and Server Actions, new hooks and APIs, and much more!
React Compiler
One of the most exciting features in React 19 is the new React Compiler, also known as React Fizz. This compiler is designed to optimize the performance of React applications by generating highly efficient JavaScript code. The React Compiler transforms your JSX and JavaScript code into highly optimized JavaScript that can execute faster, with better memory usage and less overhead. This is still in experimental mode, but it's a promising feature that will likely be a game-changer for React developers. It works with plain JavaScript, and understands the Rules of React, so you don’t need to rewrite any code to use it.
What does the compiler do?
In order to optimize the applications, React Compiler automatically memoizes the components and hooks, and it also optimizes the rendering process. This means that React will only re-render the components that have actually changed, rather than re-rendering the entire component tree. This can lead to significant performance improvements, especially for large and complex applications.
If your codebase is already very well-memoized, you might not expect to see major performance improvements with the compiler. However, in practice memoizing the correct dependencies that cause performance issues is tricky to get right by hand. The compiler can help you with that.
What does the compiler assume?
React Compiler assumes that your code:
- Is valid, semantic JavaScript
- Tests that nullable/optional values and properties are defined before accessing them (for example, by enabling
strictNullChecks
if using TypeScript), i.e.,if (object.nullableProperty) { object.nullableProperty.foo }
or with optional-chainingobject.nullableProperty?.foo
- Follows the Rules of React
React Compiler can verify many of the Rules of React statically, and will safely skip compilation when it detects an error.
Server Components and Server Actions
Server components can run at build time to read from the filesystem or fetch static content, so a web server is not required. For example, you may want to read static data from a content management system.
Server components without a server
Without Server Components, it’s common to fetch static data on the client with an Effect:
// bundle.js
import marked from 'marked'; // 35.9K (11.2K gzipped)
import sanitizeHtml from 'sanitize-html'; // 206K (63.3K gzipped)
function Page({page}) {
const [content, setContent] = useState('');
// NOTE: loads *after* first page render.
useEffect(() => {
fetch(`/api/content/${page}`).then((data) => {
setContent(data.content);
});
}, [page]);
return <div>{sanitizeHtml(marked(content))}</div>;
}
With Server Components, you can fetch static data at build time:
import marked from 'marked'; // Not included in bundle
import sanitizeHtml from 'sanitize-html'; // Not included in bundle
async function Page({page}) {
// NOTE: loads *during* render, when the app is built.
const content = await file.readFile(`${page}.md`);
return <div>{sanitizeHtml(marked(content))}</div>;
}
The rendered output can be cached and served statically, so the server doesn’t need to run any JavaScript. This can be a big win for performance, especially on mobile devices. When the app loads, it can immediately show the content, without waiting for a network request.
Server components with a server
Server Components can also run on a server. This is useful for fetching data that’s not static, like user-specific data or data that changes frequently. For example, you may want to fetch user-specific data from a database which generally is achieved by using a useEffect
hook:
// bundle.js
function Note({id}) {
const [note, setNote] = useState('');
// NOTE: loads *after* first render.
useEffect(() => {
fetch(`/api/notes/${id}`).then(data => {
setNote(data.note);
});
}, [id]);
return (
<div>
<Author id={note.authorId} />
<p>{note}</p>
</div>
);
}
function Author({id}) {
const [author, setAuthor] = useState('');
// NOTE: loads *after* Note renders.
// Causing an expensive client-server waterfall.
useEffect(() => {
fetch(`/api/authors/${id}`).then(data => {
setAuthor(data.author);
});
}, [id]);
return <span>By: {author.name}</span>;
}
With Server Components, you can read the data and render it in the component:
import db from './database';
async function Note({id}) {
// NOTE: loads *during* render.
const note = await db.notes.get(id);
return (
<div>
<Author id={note.authorId} />
<p>{note}</p>
</div>
);
}
async function Author({id}) {
// NOTE: loads *after* Note,
// but is fast if data is co-located.
const author = await db.authors.get(id);
return <span>By: {author.name}</span>;
}
Server Components can be made dynamic by re-fetching them from a server, where they can access the data and render again. This new application architecture combines the simple “request/response” mental model of server-centric Multi-Page Apps with the seamless interactivity of client-centric Single-Page Apps, giving you the best of both worlds.
Server Actions
When a Server Action is defined with the "use server"
directive, your framework will automatically create a reference to the server function, and pass that reference to the Client Component. When that function is called on the client, React will send a request to the server to execute the function, and return the result.
Server Actions can be created in Server Components and passed as props to Client Components, or they can be imported and used in Client Components.
Creating a Server Action from a Server Component:
// Server Component
import Button from './Button';
function EmptyNote () {
async function createNoteAction() {
// Server Action
'use server';
await db.notes.create();
}
return <Button onClick={createNoteAction}/>;
}
When React renders the EmptyNote
Server Component, it will create a reference to the createNoteAction
function, and pass that reference to the Button
Client Component. When the button is clicked, React will send a request to the server to execute the createNoteAction
function with the reference provided:
"use client";
export default function Button({onClick}) {
console.log(onClick);
return <button onClick={onClick}>Create Empty Note</button>
}
Importing and using a Server Action in a Client Component:
Client Components can import Server Actions from files that use the "use server"
directive:
"use server";
export async function createNoteAction() {
await db.notes.create();
}
When the bundler builds the EmptyNote
Client Component, it will create a reference to the createNoteAction
function in the bundle. When the button is clicked, React will send a request to the server to execute the createNoteAction
function using the reference provided:
"use client";
import {createNoteAction} from './actions';
function EmptyNote() {
console.log(createNoteAction);
// {$$typeof: Symbol.for("react.server.reference"), $$id: 'createNoteAction'}
<button onClick={createNoteAction} />
}
New Hooks and APIs
Besides the React Compiler and Server Components, React 19 introduces several new hooks and APIs that make it easier to build complex applications.
useOptimistic
The useOptimistic
hook allows you to update the UI optimistically before the server responds. This can make your application feel more responsive and reduce the perceived latency. The hook takes a callback function that performs the optimistic update, and an optional configuration object that specifies the server request to send after the optimistic update.
useFormStatus
The useFormStatus
hook allows you to track the status of a form, such as whether it is pristine, dirty, valid, or invalid. This can be useful for displaying feedback to the user, such as error messages or success messages.
useFormState
The useFormState
hook allows you to manage the state of a form, such as the values of the form fields and the validity of the form. This can be useful for building complex forms with dynamic validation logic.
This hook requires two arguments: the initial form state and a validation function. The validation function takes the form state as input and returns an object with the validity of each form field.
The new use
API
React 19 introduces a new use
API that is a versatile way to read values from resources like Promises or context. The use
API is similar to the useEffect
hook, but it doesn't take a callback function. Instead, it returns the value of the resource, and re-renders the component when the value changes.
const value = use(resource);
Example:
import { use } from 'react';
function MessageComponent({ messagePromise }) {
const message = use(messagePromise);
const theme = use(ThemeContext);
// ...
Conclusion - Should You Upgrade to React 19?
React 19 is a major release that introduces several groundbreaking features and enhancements to the core framework. The new React Compiler, Server Components, and Server Actions are designed to make the development process more efficient and the resulting applications faster and more powerful. The new hooks and APIs make it easier to build complex applications and improve the user experience. If you're already using React, upgrading to React 19 is definitely worth considering.
But at the same time it's important to note that React 19 is still in experimental mode, and some features may change before the final release. It's recommended to test your application with React 19 in a non-production environment before upgrading. If you're starting a new project, React 19 is a great choice, as it provides a solid foundation for building modern web applications.
Related articles
Development using CodeParrot AI
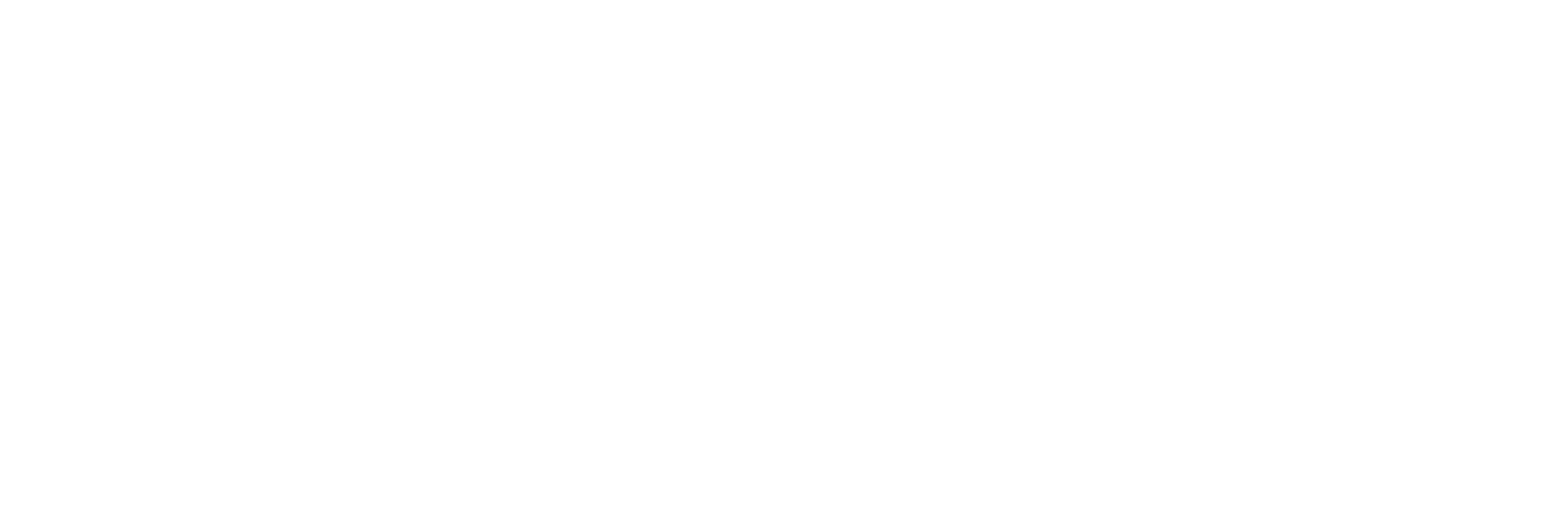