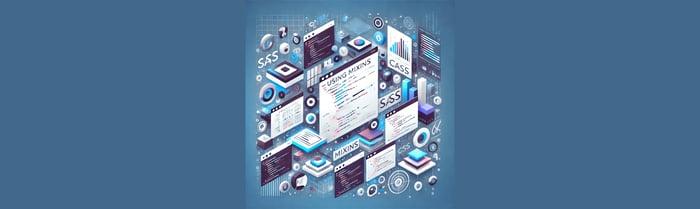
Using Mixins in Sass
If you’re someone who’s delving deeper into the world of front-end development, chances are you’ve come across Sass (Syntactically Awesome Stylesheets). Sass is a powerful CSS preprocessor that enhances your CSS workflow by providing features such as variables, nesting, functions, and mixins. Among these features, mixins stand out as a game-changer, allowing you to reuse code efficiently and maintain consistency across your stylesheets.
What are Mixins in Sass?
A mixin in Sass is a reusable block of styles that can be defined once and included wherever you need it. This eliminates the need to rewrite repetitive code, reduces redundancy, and makes your stylesheet easier to maintain. Additionally, mixins can accept parameters, making them even more powerful for dynamic styling.
Here’s a quick example of a simple mixin:
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
border-radius: $radius;
}
Now, anytime you need to apply a border-radius, you can simply include this mixin:
.button {
@include border-radius(10px);
}
Why Use Mixins?
When building complex projects, your CSS can become cluttered and repetitive. Mixins come to the rescue by enabling code reuse and parametric styling. Here are some key benefits of using mixins:
- Avoid Repetitive Code: No need to copy-paste the same CSS across multiple selectors.
- Dynamic Styling: Mixins can take parameters, allowing you to customize their behavior.
- Consistency: Ensure your styles are consistent across your entire codebase.
- Easy Maintenance: If a style needs to be updated, you only need to change it in the mixin, and it applies everywhere.
How to Create and Use Mixins
1. Defining a Simple Mixin
Here’s an example of a mixin for a box-shadow:
@mixin box-shadow($x, $y, $blur, $color) {
-webkit-box-shadow: $x $y $blur $color;
-moz-box-shadow: $x $y $blur $color;
box-shadow: $x $y $blur $color;
}
This mixin takes four parameters: horizontal and vertical offsets, blur radius, and shadow color. Now, let’s use it in a CSS class:
.card {
@include box-shadow(2px, 4px, 8px, rgba(0, 0, 0, 0.3));
}
This will generate the following CSS:
.card {
-webkit-box-shadow: 2px 4px 8px rgba(0, 0, 0, 0.3);
-moz-box-shadow: 2px 4px 8px rgba(0, 0, 0, 0.3);
box-shadow: 2px 4px 8px rgba(0, 0, 0, 0.3);
}
2. Using Default Values in Mixins
Sometimes, you may want to give your parameters default values. This way, you can use the mixin without always passing in every single argument.
@mixin transition($property: all, $duration: 0.3s, $timing-function: ease) {
transition: $property $duration $timing-function;
}
You can now call the mixin like this:
.button {
@include transition;
}
This will output:
.button {
transition: all 0.3s ease;
}
And if you want to override the default values:
.link {
@include transition(opacity, 0.5s, linear);
}
Nesting Mixins for Modular Styling
Mixins are flexible enough that you can nest mixins inside other mixins to make your code even more modular.
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
@mixin button-styles($bg-color) {
background-color: $bg-color;
padding: 10px 20px;
border: none;
color: white;
cursor: pointer;
@include flex-center;
}
Now you can apply the button-styles
mixin to multiple buttons:
.primary-button {
@include button-styles(blue);
}
.secondary-button {
@include button-styles(green);
}
Conditional Logic in Mixins
Mixins can also contain conditional logic using the @if
directive, making them even more versatile.
@mixin responsive-font-size($size) {
@if $size == small {
font-size: 12px;
} @else if $size == medium {
font-size: 16px;
} @else if $size == large {
font-size: 20px;
} @else {
font-size: 14px; // default size
}
}
You can now apply different font sizes dynamically:
.text-small {
@include responsive-font-size(small);
}
.text-large {
@include responsive-font-size(large);
}
Mixins vs. Functions: When to Use What?
Sass also provides functions, which, like mixins, allow you to encapsulate logic. So, when should you use a mixin versus a function?
- Mixins: Use when you need to generate multiple CSS declarations or apply a set of rules.
- Functions: Use when you need to return a single value, such as a color or a calculation.
For example, a function to darken a color might look like this:
@function darken-color($color, $percentage) {
@return darken($color, $percentage);
}
You’d call it like this:
$primary-color: darken-color(#3498db, 10%);
Real-World Use Cases for Mixins
Mixins are a crucial part of any real-world CSS architecture. Here are some practical scenarios where you can leverage them:
- Theming: Create a consistent design language with button, card, and typography mixins.
- Media Queries: Define media queries as mixins to make your CSS more readable and maintainable.
- Vendor Prefixing: Abstract complex vendor-prefixed properties like transitions, animations, and gradients.
- Utility Classes: Define common utilities (e.g., flexbox or grid layouts) using mixins for reuse across components.
Conclusion
Mixins in Sass are a powerful tool that can make your CSS more organized, reusable, and maintainable. By eliminating redundancy and promoting consistency, mixins help you write cleaner and more efficient stylesheets. Whether you’re working on a small project or a large application, mastering mixins will improve your development workflow.
So, next time you find yourself writing repetitive CSS, consider turning it into a mixin. Your future self (and your teammates) will thank you for it!
For more info visit the documentation.
Related articles
Development using CodeParrot AI
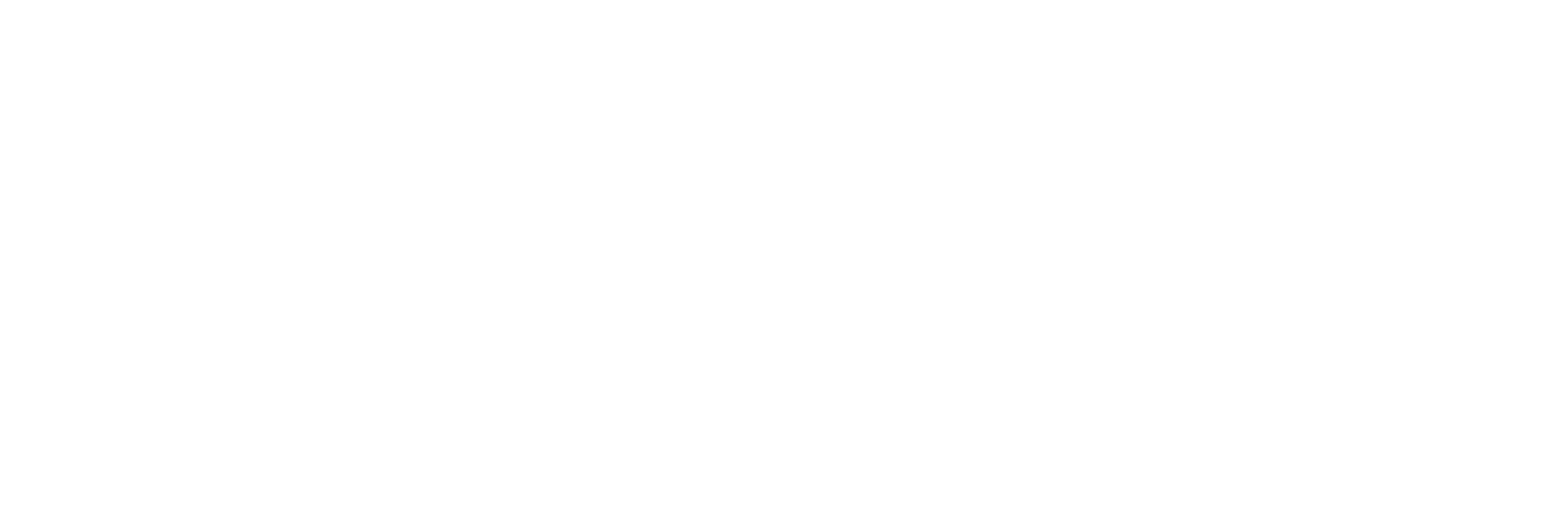