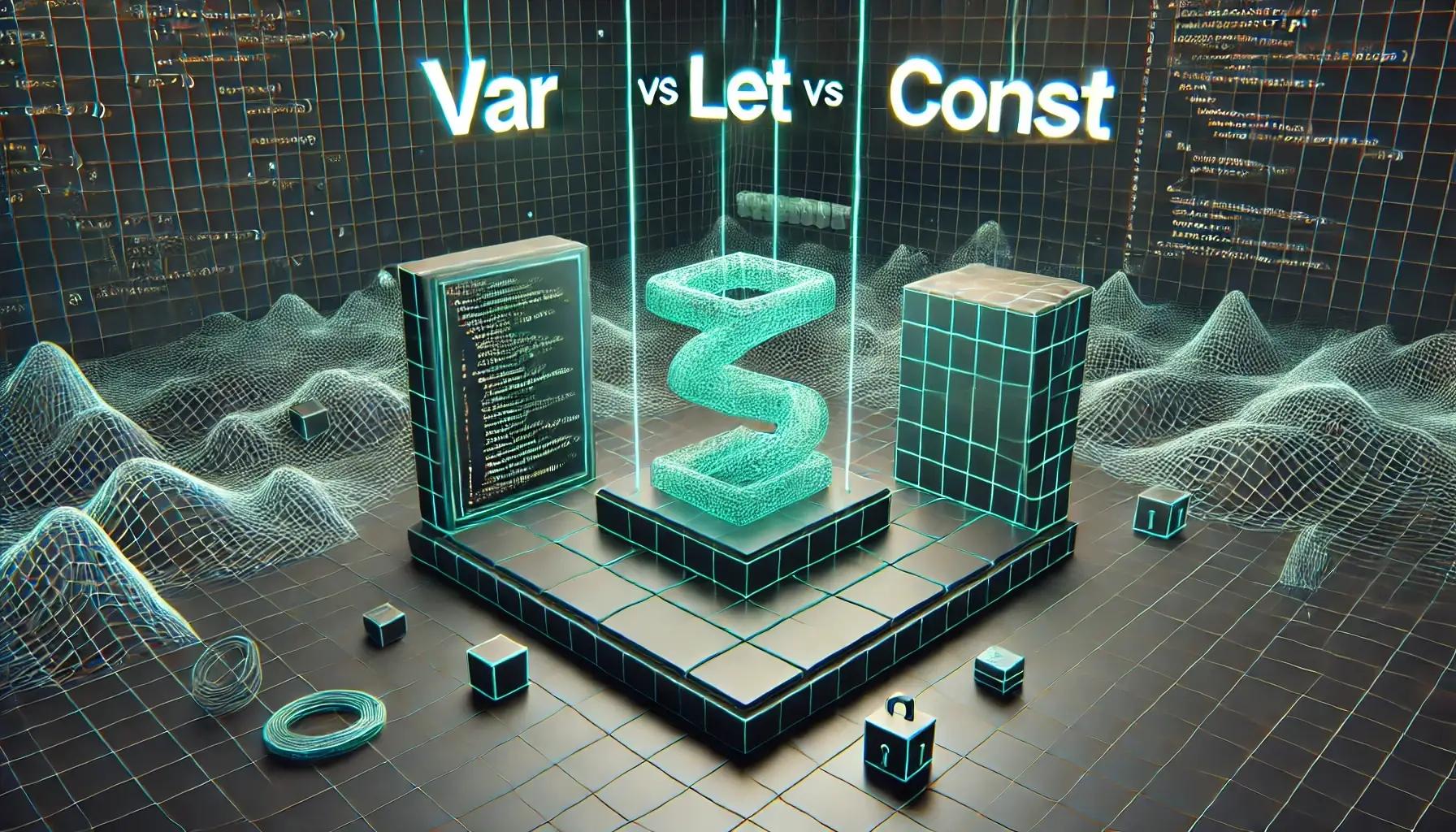
JavaScript Var vs Let vs Const: Key Differences & Best Uses
Introduction
In JavaScript, variables are fundamental building blocks that allow you to store and manipulate data throughout your code. Whether you're tracking user input, managing state, or simply holding a value for later use, variables are indispensable in any JavaScript application. As the language has evolved, so too have the methods for defining these variables. Today, the three primary ways to declare a variable are var
, let
, and const
. Understanding the differences between javascript var vs let vs const is crucial for writing clean, efficient, and bug-free code.
In this blog, we'll explore the differences between javascript var vs let vs const, compare their usage, and provide practical code examples to illustrate the best practices for each. By the end, you'll have a clear understanding of how to choose the right variable declaration for your needs, helping you write better JavaScript code.
Understanding var
var
was the original way to declare variables in JavaScript and was a staple for many years. However, as JavaScript evolved, the limitations and issues with var led to the introduction of let and const in ES6 (ECMAScript 2015). A key characteristic of var is that it is function-scoped, meaning it’s only accessible within the function where it’s declared. If declared outside a function, it becomes a global variable. This function scope differs from the block-scoping provided by let and const this is important in understanding javascript var vs let vs const.
Another important feature of var is hoisting, where variable declarations are moved to the top of their scope during execution. This allows you to reference a var variable before it is declared, but its value will be undefined until the assignment happens. While hoisting can be convenient, it often leads to confusion and subtle bugs, especially in larger codebases.
Example of Hoisting:
console.log(x); // Outputs: undefined
var x = 5;
console.log(x); // Outputs: 5
In this example, even though x
is logged before it's declared, the code doesn't throw an error. Instead, it outputs undefined
due to hoisting. JavaScript treats the code as if the var x
declaration was moved to the top of its scope.
Issues with var: Accidental Globals and Re-declaration
One of the common pitfalls with var
is the accidental creation of global variables. If you forget to use var
in a function, JavaScript will create a global variable, which can lead to unexpected behavior.
function setValue() {
value = 10; // Accidentally creates a global variable
}
setValue();
console.log(value); // Outputs: 10 (global variable created unintentionally)
Another issue is that var allows re-declaration within the same scope, which can lead to hard-to-track bugs:
var x = 10;
var x = 20;
console.log(x); // Outputs: 20
Here, the variable x is re-declared and assigned a new value, potentially overwriting the previous value without any warning.
When to Use var
In modern JavaScript let vs var vs const , var is generally discouraged in favor of let and const, which offer better scoping and prevent many common issues. However, var might still be applicable in legacy codebases where refactoring is not an option, or in certain scenarios where function-level scoping is explicitly desired.
Understanding let
let is a block-scoped variable declaration introduced in ES6 (ECMAScript 2015). Unlike var, which is function-scoped, let is confined to the block in which it is defined, such as within a loop or an if statement. This block scoping helps prevent errors and makes your code more predictable by limiting the variable's accessibility to the specific block where it is needed.
The main difference between function scope and block scope is that function-scoped variables (var) are accessible throughout the entire function in which they are declared, while block-scoped variables (let) are only accessible within the specific block, such as a loop or a conditional statement, where they are defined. This behavior of let can help avoid issues that arise from variables being unintentionally accessible outside their intended scope.
Example of let
in a Loop:
for (let i = 0; i < 3; i++) {
console.log(i); // Outputs 0, 1, 2
}
console.log(i); // ReferenceError: i is not defined
In this example, i
is only accessible within the loop due to block scoping.
Comparison with var:
if (true) {
var x = 10;
let y = 20;
}
console.log(x); // Outputs 10 (function-scoped)
console.log(y); // ReferenceError: y is not defined (block-scoped)
Here, x
is accessible outside the if
block due to var's function scope, while y
is not accessible outside the block due to let
's block scope.
Understanding const
const
is another block-scoped variable declaration introduced in ES6, similar to let
. However, const
is used to declare variables that are intended to remain constant throughout the program. The key difference between const
and let
is immutability: once a const
variable is assigned a value, it cannot be reassigned. This makes const
ideal for values that should not change, ensuring that your code is more predictable and less prone to errors.
However, it’s important to understand that const
enforces immutability on the variable binding, not the value itself. This means that while you cannot reassign a const
variable, if the value is an object or array, the contents of that object or array can still be modified.
Example with Primitive Values
const myNumber = 10;
myNumber = 20; // Error: Assignment to constant variable.
In this example, trying to reassign the value of myNumber results in an error because const does not allow reassignment.
Example with Objects/Arrays
const myArray = [1, 2, 3];
myArray.push(4); // Allowed
console.log(myArray); // Output: [1, 2, 3, 4]
const myObject = { name: "John" };
myObject.name = "Doe"; // Allowed
console.log(myObject); // Output: { name: "Doe" }
Here, even though the myArray
and myObject
variables are declared with const, their contents can be modified. The const
keyword only ensures that the variable itself cannot be reassigned, not that the data inside the object or array is immutable.
When to Use const
Best practices in modern JavaScript suggest using const
by default for most variables. This approach helps prevent unintended variable reassignment and makes your code more reliable. You should only use let
when you know that a variable's value will need to be reassigned. By adhering to this principle, you can reduce bugs and improve the overall quality of your code.
Comparing var, let, and const
Key Differences:
Feature | var | let | const |
---|---|---|---|
Scope | Function-scoped | Block-scoped | Block-scoped |
Hoisting | Hoisted (initialized as undefined ) | Hoisted (but not initialized) | Hoisted (but not initialized) |
Re-declaration | Allowed within the same scope | Not allowed in the same scope | Not allowed in the same scope |
Immutability | Mutable | Mutable | Immutable binding, but mutable contents for objects/arrays |
Code Examples
Example of Scope:
function scopeTest() {
if (true) {
var a = 1;
let b = 2;
const c = 3;
}
console.log(a); // Outputs 1 (function-scoped)
console.log(b); // ReferenceError: b is not defined (block-scoped)
console.log(c); // ReferenceError: c is not defined (block-scoped)
}
scopeTest();
In this example, var
is function-scoped, so a
is accessible outside the if block. However, let
and const
are block-scoped, so b
and c
are not accessible outside the block they were defined in.
Example of Hoisting:
console.log(varVar); // Outputs undefined
console.log(letVar); // ReferenceError: Cannot access 'letVar' before initialization
console.log(constVar); // ReferenceError: Cannot access 'constVar' before initialization
var varVar = "var";
let letVar = "let";
const constVar = "const";
Here, var
is hoisted and initialized as undefined
, so it can be referenced before its declaration without causing an error. However, in javascript var vs let vs const , let
and const
are hoisted but not initialized, resulting in a ReferenceError
if accessed before their declarations.
Example of Re-declaration
var x = 20; // No error, x is now 20
let y = 10;
let y = 20; // Error: Identifier 'y' has already been declared
const z = 10;
const z = 20; // Error: Identifier 'z' has already been declared
With var
, re-declaring the same variable is allowed, and the value is updated. However,among javascript var vs let vs const let
and const
do not allow re-declaration within the same scope, leading to an error if you try to do so.
Example of Immutability:
myArray.push(4); // Allowed
console.log(myArray); // Output: [1, 2, 3, 4]
myArray = [4, 5, 6]; // Error: Assignment to constant variable
In this case, const prevents the reassignment of the variable myArray, which would result in an error. However, the contents of the array can still be modified, such as adding a new element.
Best Practices
In modern JavaScript var vs let vs const, the consensus among developers is to use const
and let
in place of var to ensure code that is more predictable, maintainable, and less prone to bugs. Here are some best practices to follow:
- Use
const
by Default Whenever possible, useconst
to declare variables. Sinceconst
ensures that the variable cannot be reassigned, it makes your code easier to understand and prevents accidental modifications. By defaulting toconst
, you signal to other developers (and yourself) that the value should remain constant throughout the code's execution. - Use let Only When Reassignment is Necessary If you know that a variable's value will need to change, use
let
.let
allows for reassignment while still providing the benefits of block-scoping, which helps avoid issues that can arise from variables leaking out of their intended scope. - Avoid var in Modern JavaScript In modern JavaScript var vs let vs const topic, it’s best to avoid using
var
altogether. var's function-scoping, hoisting and the ability to be redeclared can lead to unpredictable behavior, especially in larger codebases. The only time you might need to usevar
is when maintaining or working with legacy code that relies on it. - Sample Refactor: Converting var to let and const Here’s a simple example of refactoring older JavaScript code that uses var to a more modern approach with let and const
Before Refactoring:
After Refactoring:function calculateTotal(prices) { var total = 0; for (var i = 0; i < prices.length; i++) { var price = prices[i]; total += price; } var discount = 0.1; var finalTotal = total - (total * discount); return finalTotal; }
In the refactored version, the total is declared with let since its value changes throughout the function. price, discount, and finalTotal are declared with const because their values are not reassigned after their initial assignment. This refactoring makes the function more robust and easier to reason about, reducing the likelihood of accidental errors when using javascript var vs let vs const.function calculateTotal(prices) { let total = 0; for (let i = 0; i < prices.length; i++) { const price = prices[i]; // price doesn't change within the loop total += price; } const discount = 0.1; // discount remains constant const finalTotal = total - (total * discount); // finalTotal doesn't change after calculation return finalTotal; }
Common Pitfalls and How to Avoid Them
When working with javascript var vs let vs const, developers often encounter common pitfalls that can lead to bugs or unexpected behavior. Understanding these pitfalls and knowing how to avoid them is crucial for writing clean, reliable code.
Accidental Global Variables with var
One of the most common mistakes with var
is accidentally creating global variables. This happens when a var
declaration is omitted inside a function or block, causing the variable to be attached to the global object.
function calculate() {
total = 100; // No var/let/const declaration, creates a global variable
}
calculate();
console.log(total); // Outputs 100, but total is now global!
How to Avoid: Always use let
or const
to declare variables. This ensures that the variable is scoped to the block or function in which it is defined, preventing unintended global variables.
Hoisting Confusion with var
var
is hoisted to the top of its scope, but only the declaration is hoisted, not the assignment. This can lead to confusing behavior if you try to use the variable before it is assigned.
console.log(name); // Outputs undefined
var name = "Alice";
How to Avoid: Use let
or const
, which are also hoisted but not initialized. This prevents variables from being accessed before they are defined, reducing the chance of errors.
Re-declaration with var
var
allows for re-declaration within the same scope, which can lead to unexpected overwrites and bugs, especially in larger functions.
var count = 10;
var count = 20; // No error, but original value is lost
How to Avoid: Avoid using var
. Among javascript var vs let vs const , Use let
or const
instead, which do not allow re-declaration within the same scope. This ensures that variable names are unique and helps prevent accidental overwrites.
Misunderstanding const
with Objects and Arrays
Many developers assume that const
makes the entire object or array immutable, but in reality, it only prevents reassignment of the variable. The contents of the object or array can still be modified.
const person = { name: "Alice" };
person.name = "Bob"; // Allowed, object properties can be modified
person = { name: "Charlie" }; // Error: Assignment to constant variable
How to Avoid: Understand that const
applies to the variable binding, not the value itself. If you need a truly immutable object or array, consider using methods like Object.freeze()
or libraries that enforce immutability.
Scope Misconceptions with let
and const
Developers may incorrectly assume that variables declared with let
or const
are accessible outside of the block they were defined in, similar to var
.
if (true) {
let x = 10;
}
console.log(x); // ReferenceError: x is not defined
Always be aware of the block scope when using let and const. If you need a variable to be accessible in a wider scope, declare it outside the block.
By understanding these common pitfalls and using var
, let
, and const
appropriately, you can avoid many of the issues that commonly arise in JavaScript development. This leads to cleaner, more maintainable, and less error-prone code.
Conclusion
In this blog, we've explored the key differences between javascript var vs let vs const—the three primary ways to define variables in JavaScript. We've seen how var is function-scoped and hoisted, but its quirks can lead to unintended behavior. On the other hand, let and const, introduced in ES6, offer block-scoping and greater predictability, making them the preferred choices for modern JavaScript development.
For further reading and to deepen your understanding of JavaScript var vs let vs const, check out the following resources:
Understanding when and how to use javascript var vs let vs const is crucial for writing clean, efficient, and bug-free code. By defaulting to const, using let only when necessary, and avoiding var in new code, you can avoid many common pitfalls and improve the maintainability of your projects.
Related articles
Development using CodeParrot AI
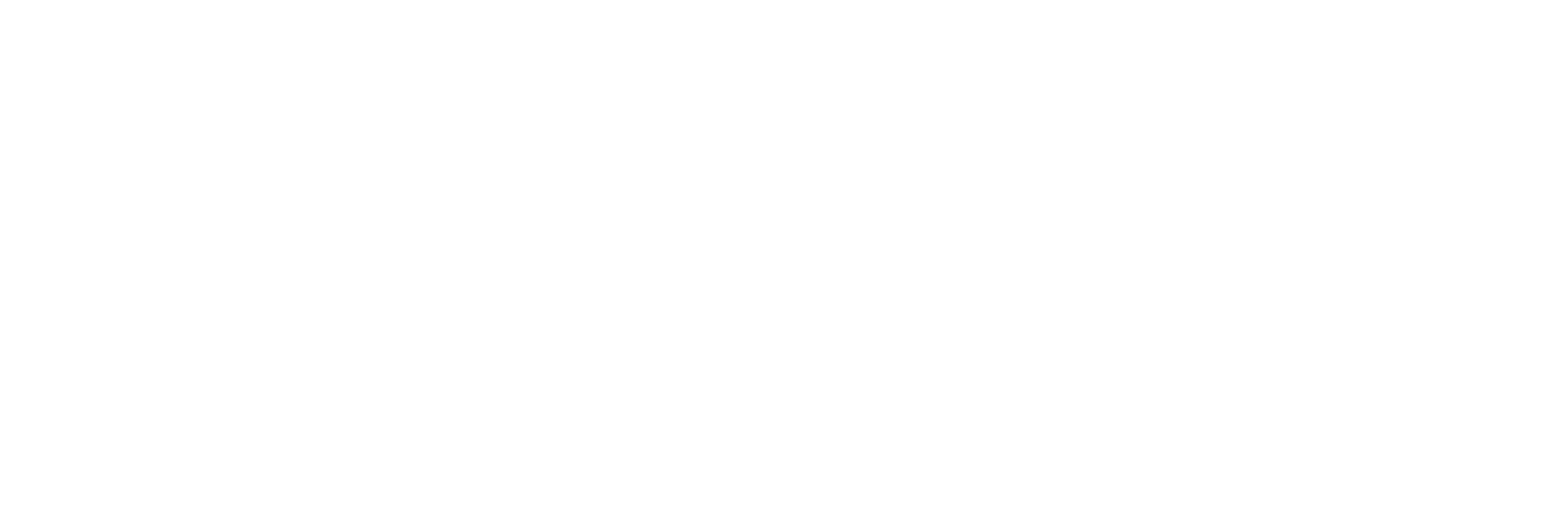