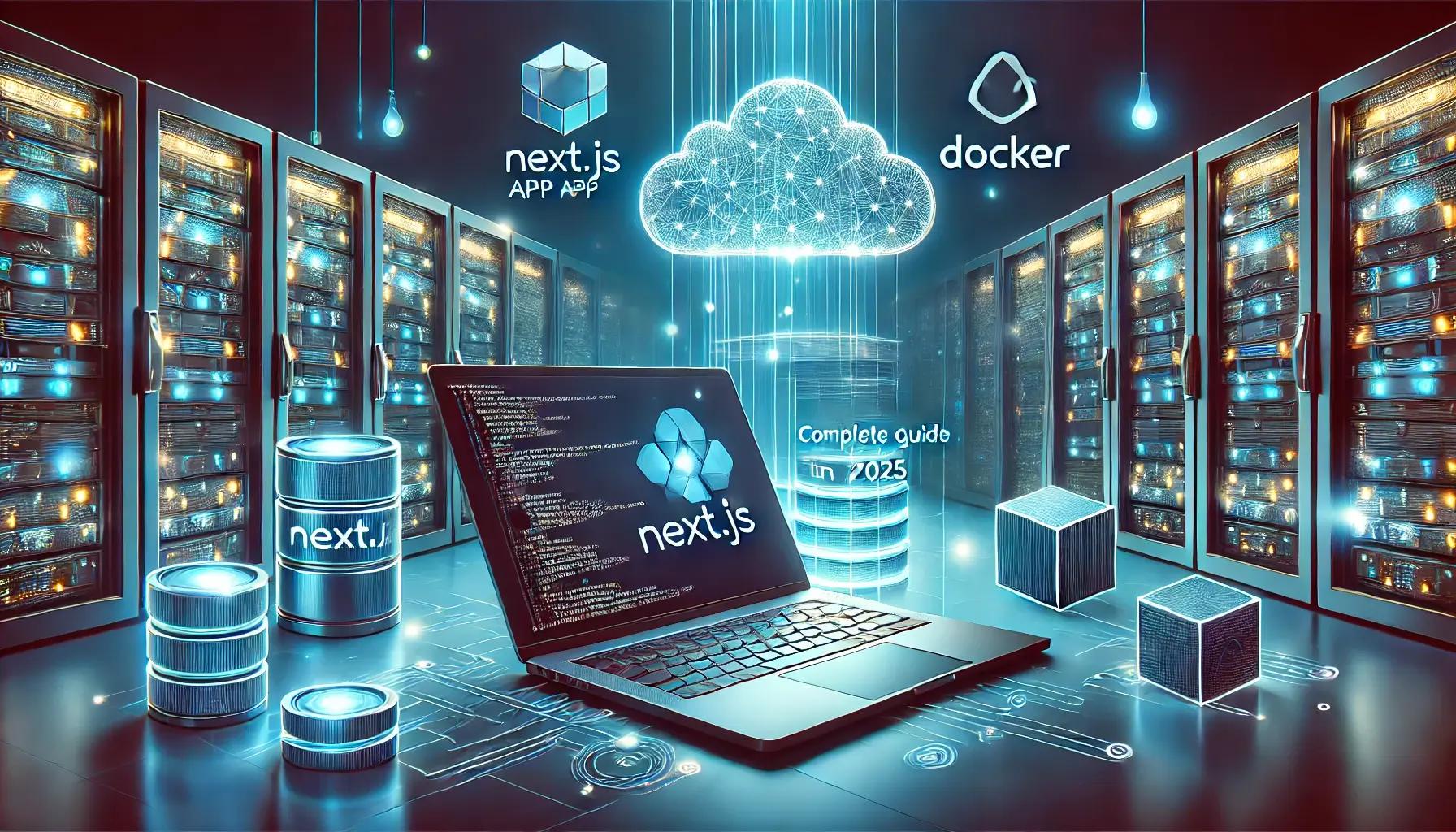
NextJs App Deployment with Docker: Complete Guide for 2025
Containerizing your NextJs application with Docker in 2025 simplifies deployments by ensuring consistency across all environments—whether local development, testing, or production. Docker packages your app with all its dependencies, providing portability across platforms like Railway, AWS EC2, ECR, and beyond, eliminating common deployment headaches like version conflicts or environment mismatches.
Using Docker also means seamless deployments across cloud platforms—Railway effortlessly deploys Dockerized apps directly from your GitHub repository, while AWS leverages Elastic Container Registry (ECR) and EC2 for robust, scalable infrastructure. In this guide, we'll walk through deploying your Next.js application using Docker, comparing it briefly to other deployment methods, and sharing best practices for optimal performance and security.
Setting Up Docker for Next.js
To containerize your Next.js app effectively, you'll first need Docker installed on your machine. You can easily get Docker Desktop for Windows, macOS, or Linux from Docker's official website. After installation, confirm it's ready by typing docker --version
in your terminal—you should see a version number, indicating everything's set.
Next, create a Dockerfile in your Next.js project's root directory. This Dockerfile instructs Docker on how to package your app, dependencies, and environment into a container. We'll use a multi-stage build process, optimizing for production by keeping the image lightweight and efficient. Here's a simplified yet robust Dockerfile example to start with:
FROM node:22-alpine AS builder
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
RUN npm run build
FROM node:22-alpine AS runner
WORKDIR /app
COPY --from=builder /app ./
EXPOSE 3000
CMD ["npm", "start"]
Let’s briefly decode the Dockerfile:
FROM node:22-alpine
: Uses the lightweight Alpine Linux version of Node.js 22 for stability and minimal image size.- Multi-stage build: Separates the app's build (first stage) from its runtime (second stage), keeping your final Docker image lean and efficient.
npm run build
ensures your Next.js app compiles correctly into the.next
directory for production.- Finally, the
EXPOSE
instruction indicates port 3000 (Next.js default), and the container launches your app usingnpm start
.
After setting this up, build and test your Docker image locally with:
docker build -t my-nextjs-app .
docker run -p 3000:3000 my-nextjs-app
Visit http://localhost:3000
in your browser. You should see your Next.js application running smoothly—exactly as it will run anywhere else Docker is supported.
3. Deploying Next.js with Docker on Railway
Railway is a cloud platform that makes Docker deployments exceptionally easy, automatically detecting your Dockerfile and building your Next.js application directly from your GitHub repository. Here’s how you can quickly deploy your containerized Next.js app on Railway: First, log in to Railway and select "New Project". Choose the option "Deploy from GitHub Repo", connect your GitHub account if needed, and pick the repository containing your Next.js app and Dockerfile.
Railway automatically detects your Dockerfile and initiates the build, requiring no extra configuration if the Dockerfile is placed in your project's root.
Once deployment is complete, Railway provides a public domain (your-app.up.railway.app
) where your Next.js application is live. Any new commits pushed to your GitHub repository automatically trigger a new deployment. Railway also manages environment variables effortlessly, which you can set directly from the Railway dashboard, simplifying management of secrets and configs. If something goes wrong, detailed deployment logs are accessible via the Railway interface, making troubleshooting straightforward.
Deploying Next.js with Docker on AWS (ECR & EC2)
Deploying your Dockerized Next.js application on AWS involves two straightforward steps: storing your Docker image in Amazon’s Elastic Container Registry (ECR) and running it on an EC2 instance.
Pushing the Docker Image to AWS ECR
First, log in to your AWS console and navigate to Elastic Container Registry (ECR). Create a new repository, like nextjs-app
. Note down the provided repository URI (aws_account_id.dkr.ecr.region.amazonaws.com/nextjs-app
).
Authenticate Docker to your ECR using the AWS CLI. Run the following in your terminal:
aws ecr get-login-password --region <your-region> | docker login --username AWS --password-stdin aws_account_id.dkr.ecr.<region>.amazonaws.com
Now build and tag your Docker image for ECR:
docker build -t nextjs-app .
docker tag nextjs-app:latest aws_account_id.dkr.ecr.<region>.amazonaws.com/nextjs-app:latest
docker push aws_account_id.dkr.ecr.<region>.amazonaws.com/nextjs-app:latest
Your image is now securely stored in ECR and ready to deploy.
Running the Next.js Container on EC2
Launch an EC2 instance (like Ubuntu or Amazon Linux) and install Docker:
sudo yum update -y
sudo yum install docker -y
sudo service docker start
Authenticate Docker on EC2 to pull your image from ECR:
aws ecr get-login-password --region <region> | docker login --username AWS --password-stdin aws_account_id.dkr.ecr.<region>.amazonaws.com
Pull and run your container on EC2:
docker pull aws_account_id.dkr.ecr.<region>.amazonaws.com/nextjs-app:latest
docker run -d -p 80:3000 --restart unless-stopped aws_account_id.dkr.ecr.<region>.amazonaws.com/nextjs-app:latest
Your Next.js app is now live at your EC2’s public IP. Remember to configure your EC2 Security Group to allow inbound traffic on the app’s port (typically port 80 or 3000).
Docker Deployment vs. Other Methods: A Quick Comparison
Deploying Next.js applications with Docker gives you a lot of flexibility and control. Compared to managed hosting services like Vercel—which offer out-of-the-box deployments optimized specifically for Next.js apps—Docker gives you greater customization and portability across various cloud platforms. Docker deployments ensure consistency across environments, whether you're deploying to Railway, AWS, Azure, or running locally, reducing the friction often associated with traditional hosting setups.
On the other hand, platforms like Vercel offer zero-configuration deployments, automatic scalability, and built-in optimizations specifically tailored for Next.js. However, this ease comes with reduced control over infrastructure and potentially higher costs as your project grows. Docker strikes a balance, offering control, consistency, and scalability across diverse platforms, making it ideal for teams who value portability, flexibility, and consistency across various deployment environments.
Troubleshooting and Best Practices
When deploying your Dockerized Next.js application, you may occasionally encounter common challenges. One frequent issue is the error: "Could not find a production build in the .next
directory". This usually means your Dockerfile missed the build step (npm run build
). Always ensure your Dockerfile explicitly runs the Next.js build command before starting the app.
Another common pitfall is networking configuration, particularly with AWS EC2. If your application isn’t accessible, double-check the security group rules to confirm the correct ports (typically 80
or 3000
) are open. Also, verify that Next.js listens on all network interfaces (0.0.0.0
) rather than localhost alone.
Best Practices:
- Use multi-stage builds: Keep Docker images lean by copying only essential files from the build stage.
- .dockerignore: Exclude unnecessary files (
node_modules
, test files, secrets) to speed up Docker builds. - Environment variables: Manage sensitive configuration through runtime environment variables rather than embedding them directly in the image.
- Logging and Monitoring: Regularly check logs via
docker logs <container-id>
or your platform's built-in logging (Railway, AWS CloudWatch) to quickly diagnose issues and maintain reliability.
Performance Optimizations & Security Best Practices
When deploying your Dockerized Next.js application, consider the following best practices to enhance performance and security:
Performance Optimizations:
- Use Multi-stage Builds: Keep your Docker images lightweight by copying only essential build artifacts, significantly reducing build and deployment times.
- Efficient Docker Layers: Structure your Dockerfile so less-frequently changed files (like dependencies) are copied first, leveraging caching and reducing build times.
- Minimal Base Images: Use lightweight images like
node:22-alpine
to reduce container size and improve startup performance.
Security Best Practices:
- Run as Non-root User: Always run your Next.js application within the container using a non-root user to minimize risks if your container gets compromised.
- Secrets Management: Store sensitive configurations (API keys, database credentials) as environment variables or managed secrets rather than embedding them in Docker images.
- Regularly Update Base Images: Keep your base images updated to apply the latest security patches and minimize vulnerabilities.
- Restrict Network Access: Configure your cloud provider's firewall rules (such as AWS Security Groups) to allow traffic only on essential ports, minimizing the attack surface.
Conclusion
Deploying your Next.js application with Docker in 2025 is a reliable and flexible approach, enabling seamless deployments to platforms like Railway and AWS (ECR and EC2). By containerizing your app, you ensure consistency across environments, simplify deployments, and easily scale when needed.
By adopting Docker, you can seamlessly deploy to platforms such as Railway and leverage AWS services like Elastic Container Registry (ECR) and Elastic Compute Cloud (EC2). Docker also integrates smoothly with modern CI/CD workflows and Infrastructure as Code, aligning perfectly with DevOps best practices.
Whether you're managing personal projects or enterprise-level apps, Dockerizing Next.js provides a robust foundation for scalable, secure, and consistent deployments.
Useful Links:
Related articles
Development using CodeParrot AI
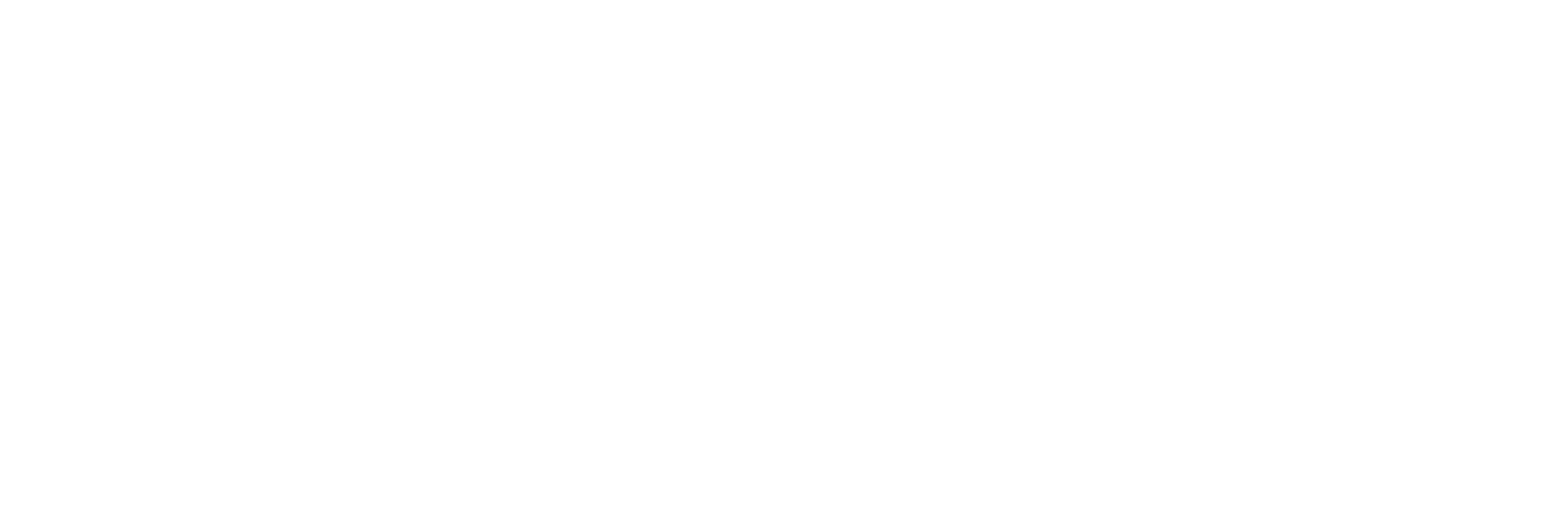