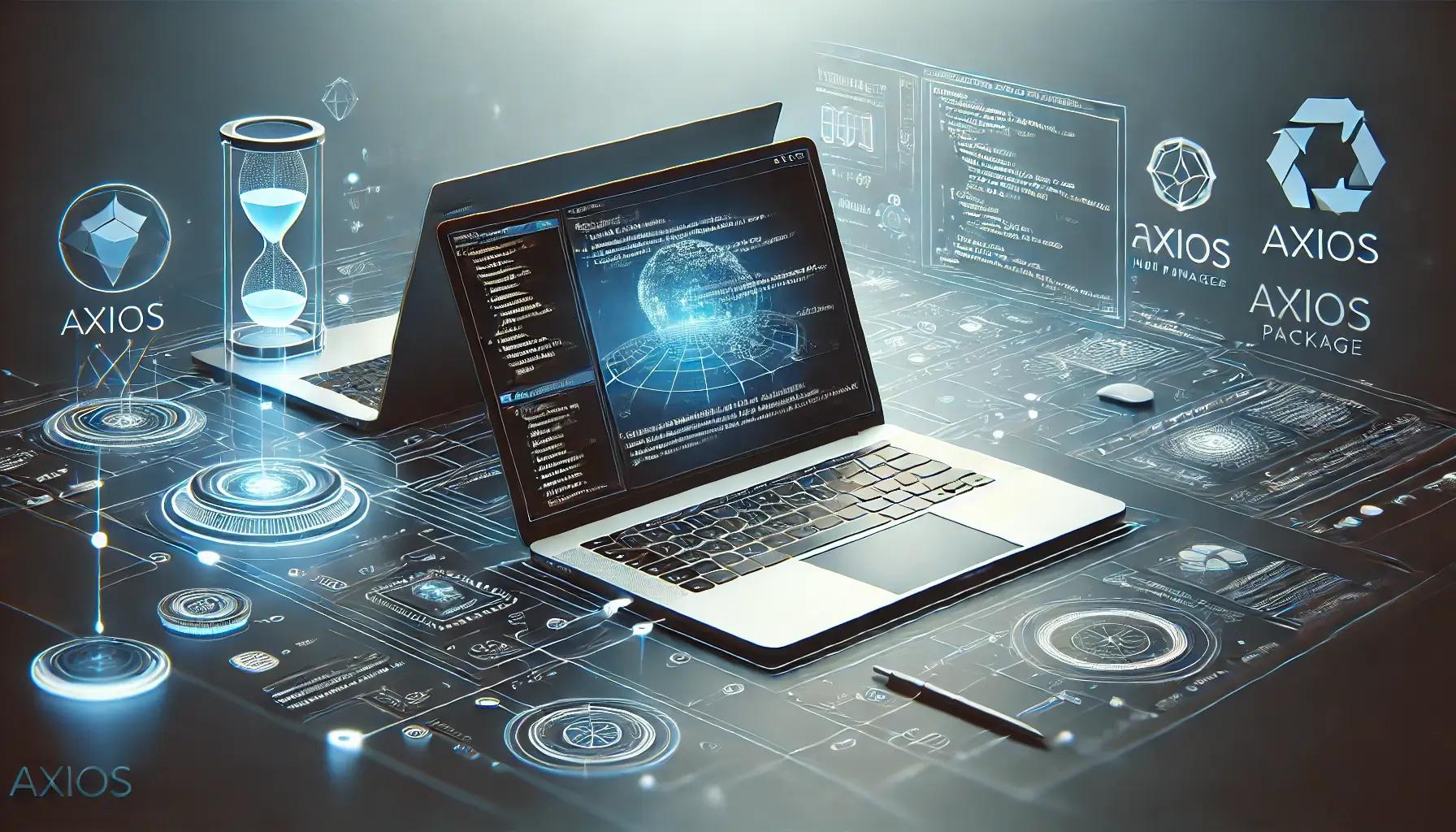
Axios NPM Package: A Beginner's Guide to Installing and Making HTTP Requests
Introduction
When building modern web applications, making HTTP requests is a core task for fetching or sending data to a server. While JavaScript provides the fetch
API as a native way to handle these requests, many developers prefer using Axios npm package, a feature-rich and intuitive library. Axios simplifies the process by offering a promise-based HTTP client that works seamlessly in both browsers and Node.js environments. Its support for async/await
makes code easier to read and maintain, especially when handling multiple requests.
This blog will help you get started with Axios npm package, covering how to install it and use it for basic HTTP operations like GET
, POST
, and PUT
. We'll also dive into its features and why it's a go-to choice for developers over alternatives like the native fetch
API.
What is Axios?
Axios is a lightweight JavaScript library designed to make HTTP requests simpler and more efficient. It operates as a promise-based client, allowing developers to handle asynchronous data flow in a cleaner and more manageable way. Whether you’re working in the browser or in a Node.js environment, Axios provides a unified solution for interacting with APIs.
Features of Axios
- Promise-based: Works seamlessly with promises and supports
async/await
syntax for cleaner asynchronous code. - Automatic JSON Transformation: Axios automatically converts JSON responses to JavaScript objects, saving the extra step of parsing the data manually.
- Request and Response Interceptors: It allows developers to modify requests or responses globally before they are handled.
- Default Configurations: You can create Axios instances with predefined configurations like base URLs or headers, reducing repetitive code.
- Error Handling: Provides robust error handling with detailed error messages, making debugging easier.
Why Choose Axios Over fetch?
While the fetch
API is natively available in JavaScript, Axios offers several advantages that make it a preferred choice:
- Automatic JSON Handling: With
fetch
, developers need to manually parse the response usingresponse.json()
. Axios does this automatically.// Using fetch fetch(url) .then(res => res.json()) .then(data => console.log(data)); // Using Axios axios.get(url) .then(response => console.log(response.data));
- Request Interceptors: Axios enables developers to modify headers or handle authentication tokens through interceptors, which is not natively supported by
fetch
. - Error Handling: Axios provides a more detailed error object, while
fetch
considers HTTP response codes like 404 or 500 as successful requests unless explicitly checked. - Support for Older Browsers: Axios includes built-in support for older browsers, unlike
fetch
, which may require polyfills.
These features, combined with its ease of use, make Axios npm a reliable and developer-friendly tool for handling HTTP requests.
If you're interested in a more in-depth comparison, we have another blog that dives deeper into the nuances of Axios vs fetch, discussing when to choose one over the other. Check it out here: Axios vs Fetch: Which One Should You Choose for Your Project?.
How to Install Axios npm
Getting started with Axios npm is quick and easy. Below are the step-by-step instructions for installing and including Axios in your project.
Step 1: Installing Axios
To use Axios, you first need to install it in your project. You can do this using either npm
or yarn
.
Using npm (Node Package Manager): Open your terminal in the project directory and run the following command:
npm install axios
Using Yarn: If you’re using Yarn as your package manager, run:
yarn add axios
This will add Axios as a dependency to your package.json
file.
Step 2: Including Axios in Your Project
After installing Axios, you need to import it into your JavaScript or TypeScript file.
Using CommonJS Syntax: If you’re working in a CommonJS environment (e.g., Node.js), you can include Axios using
require
:const axios = require('axios');
Using ES6 Syntax: For ES6 modules or modern JavaScript frameworks like React, import Axios as follows:
import axios from 'axios';
Both approaches will work depending on your project setup and JavaScript environment.
Verifying Installation
Here’s a simple code snippet to verify that Axios has been installed and imported correctly:
import axios from 'axios';
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log('Axios is working:', response.data);
})
.catch(error => {
console.error('Error using Axios:', error);
});
Run this code in your project, and if you see the fetched data logged in your console, you’ve successfully installed and included Axios npm in your project.
4. Understanding HTTP Methods with Axios
Axios makes handling HTTP methods like GET
, POST
, PUT
, and DELETE
straightforward with its intuitive syntax. Let’s explore each of these methods in detail, with examples demonstrating how to use them.
4.1. GET Request
A GET
request is used to retrieve data from a server. This is one of the most common HTTP methods, typically used to fetch lists, user profiles, or any read-only data.
Code Example:
axios.get('https://jsonplaceholder.typicode.com/users')
.then(response => console.log(response.data))
.catch(error => console.error(error));
Explanation:
axios.get(url)
sends aGET
request to the provided URL.- The
response.data
contains the data fetched from the server. - The
.catch()
block handles any errors, such as network issues or invalid endpoints.
Example Output:
[
{ "id": 1, "name": "Leanne Graham", "email": "leanne@example.com" },
{ "id": 2, "name": "Ervin Howell", "email": "ervin@example.com" }
]
4.2. POST Request
A POST
request is used to send data to a server, typically for creating new records like user registrations or blog posts.
Code Example:
axios.post('https://jsonplaceholder.typicode.com/posts', {
title: 'New Post',
body: 'This is a new post.',
userId: 1
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Explanation:
axios.post(url, data)
sends aPOST
request to the server with the data specified in the second argument.- In this example, the request sends a new post with a
title
,body
, anduserId
. - The response from the server includes the newly created resource.
Example Output:
{
"id": 101,
"title": "New Post",
"body": "This is a new post.",
"userId": 1
}
4.3. PUT Request
A PUT
request is used to update an existing resource. It typically replaces the entire resource with the updated data.
Code Example:
axios.put('https://jsonplaceholder.typicode.com/posts/1', {
id: 1,
title: 'Updated Post',
body: 'This post has been updated.',
userId: 1
})
.then(response => console.log(response.data))
.catch(error => console.error(error));
Explanation:
axios.put(url, data)
sends aPUT
request to update the resource at the given URL.- The second argument contains the updated data, which in this case updates the
title
andbody
of the post withid: 1
. - The server responds with the updated resource.
Example Output:
{
"id": 1,
"title": "Updated Post",
"body": "This post has been updated.",
"userId": 1
}
4.4. DELETE Request
A DELETE
request is used to remove a resource from the server. It’s commonly used for deleting records such as user profiles or posts.
Code Example:
axios.delete('https://jsonplaceholder.typicode.com/posts/1')
.then(response => console.log('Deleted:', response.data))
.catch(error => console.error(error));
Explanation:
axios.delete(url)
sends aDELETE
request to the server.- The server removes the resource specified by the URL (
/posts/1
in this case) and may return a confirmation response.
Example Output:
{}
An empty response indicates that the deletion was successful.
With these HTTP methods, Axios provides a clean and concise way to interact with APIs for all CRUD (Create, Read, Update, Delete) operations. Its promise-based structure and robust error handling make it a powerful tool for any project. Let’s now explore some advanced features of Axios!
Advanced Features of Axios
While Axios is straightforward for basic HTTP requests, it also offers advanced features that make it a powerful tool for more complex use cases. Here are some of its notable advanced features:
Configuring Headers
- Axios allows you to customize request headers, which is particularly useful for sending authentication tokens or setting content types.
- Example of setting a header for an API request:
axios.get('https://api.example.com/data', { headers: { Authorization: 'Bearer your-auth-token', 'Content-Type': 'application/json' } }) .then(response => console.log(response.data)) .catch(error => console.error(error));
Setting Up Axios Instances
- If you’re working with an API that requires repetitive configurations, you can create an Axios instance with predefined settings like base URLs and default headers.
- Example of creating an Axios instance:
const apiClient = axios.create({ baseURL: 'https://api.example.com', headers: { 'Content-Type': 'application/json', Authorization: 'Bearer your-auth-token' } }); // Using the instance for a GET request apiClient.get('/endpoint') .then(response => console.log(response.data)) .catch(error => console.error(error));
Using Interceptors
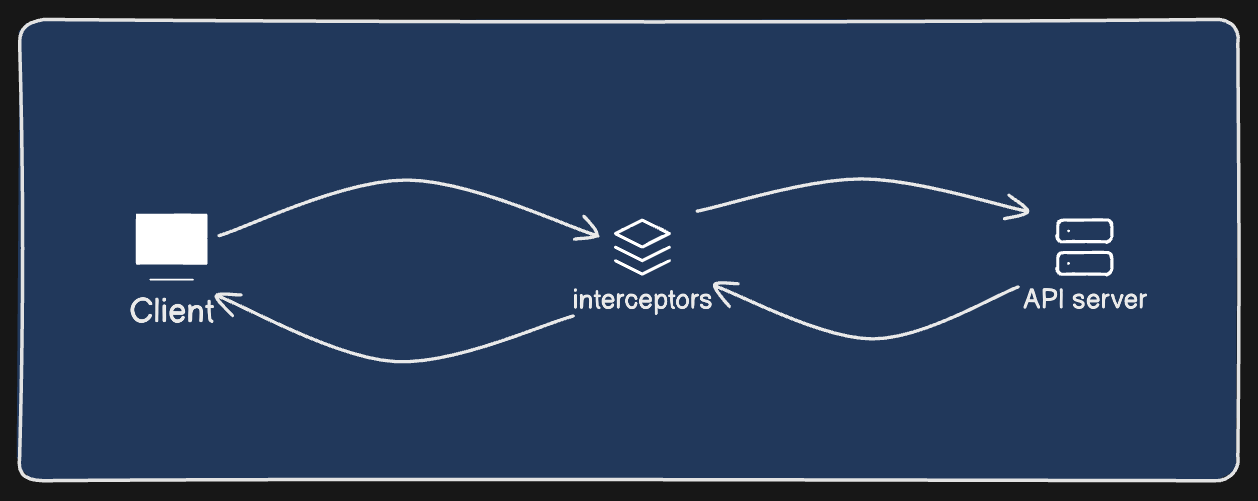
- Interceptors allow you to modify requests or responses globally before they are handled. This is useful for adding headers, logging requests, or handling errors in a centralized way
- Example of a request interceptor:
axios.interceptors.request.use( config => { console.log('Request sent:', config); // Add custom logic, like appending an auth token config.headers.Authorization = 'Bearer your-auth-token'; return config; }, error => { return Promise.reject(error); } ); // Example of a response interceptor axios.interceptors.response.use( response => { console.log('Response received:', response); return response; }, error => { console.error('Error occurred:', error); return Promise.reject(error); } );
With these advanced features, you can optimize your Axios usage for better performance, scalability, and maintainability in your applications.
Troubleshooting Common Issues
Like any tool, using Axios npm may come with challenges. Here are some common issues developers face and how to resolve them:
CORS Issues
- Problem: Cross-Origin Resource Sharing (CORS) errors occur when the API server does not allow requests from your domain.
- Solution:
- Ensure the server supports CORS by enabling proper headers like
Access-Control-Allow-Origin
. - Use a proxy or browser extension during development to bypass the error.
- Ensure the server supports CORS by enabling proper headers like
Request Timeout
- Problem: The API server takes too long to respond, causing a timeout.
- Solution:Set a timeout in your Axios request configuration:
axios.get('https://api.example.com/data', { timeout: 5000 }) // 5 seconds .then(response => console.log(response.data)) .catch(error => { if (error.code === 'ECONNABORTED') { console.error('Request timed out'); } else { console.error('Error:', error); } });
Network Errors
- Problem: Issues like
ENOTFOUND
orERR_NETWORK
occur due to connectivity problems. - Solution:Check your network connection and API URL. Add retry logic for transient errors:
async function fetchDataWithRetry(url, retries = 3) { for (let i = 0; i < retries; i++) { try { const response = await axios.get(url); return response.data; } catch (error) { if (i === retries - 1) throw error; } } }
Debugging Errors
- Problem: Axios errors may not always be self-explanatory.
- Solution:Check the error object for details:
axios.get('https://api.example.com/data') .catch(error => { console.error('Error message:', error.message); console.error('Error config:', error.config); console.error('Error response:', error.response); });
Unhandled Promise Rejection
- Problem: Forgetting to handle
.catch()
can lead to unhandled promise rejection warnings. - Solution: Always include a
.catch()
block or usetry/catch
withasync/await
to manage errors.
By addressing these common issues, you can ensure a smoother experience while working with Axios npm in your projects.
Conclusion
In this guide, we’ve explored the fundamentals of using Axios npm for making HTTP requests in JavaScript. From installing Axios to creating your first GET
, POST
,PUT
and DELETE
requests, you’ve seen how it simplifies the process with its promise-based structure, automatic JSON parsing, and robust error-handling features. We also touched on advanced capabilities like configuring headers, creating reusable Axios instances, and using interceptors for request/response modification.
Axios is a powerful tool that can streamline how you handle API requests in your projects. Whether you’re building a simple web application or managing complex API integrations, Axios makes the process intuitive and efficient.
If you haven’t already, give Axios npm a try in your own projects and experience the ease of handling HTTP requests. For more detailed information and examples, explore the official Axios documentation and take your skills to the next level!
Related articles
Development using CodeParrot AI
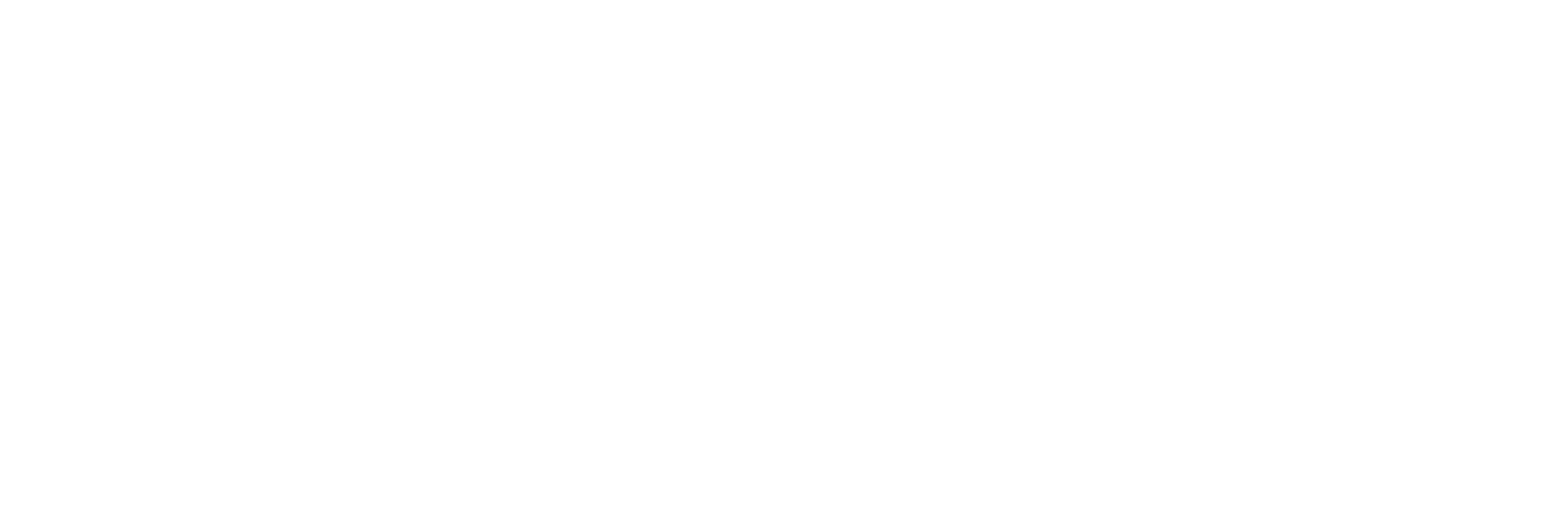