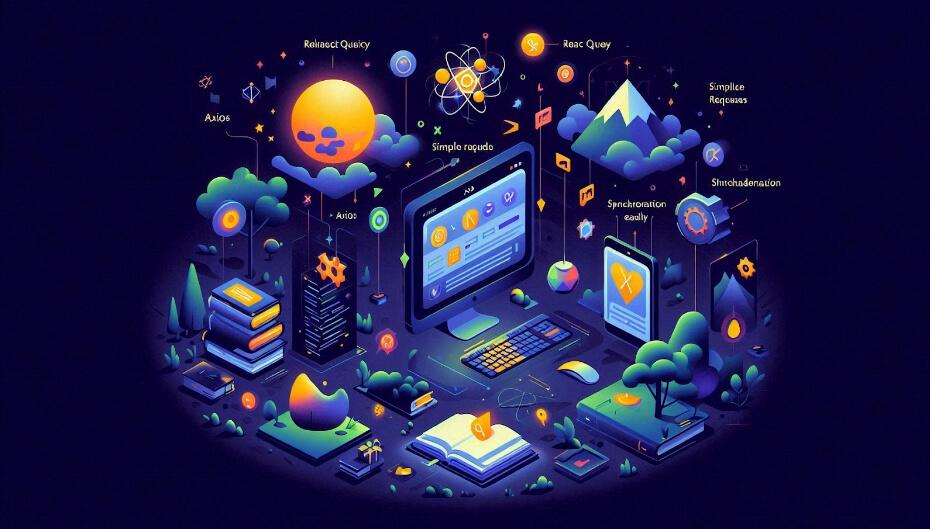
React Query vs Axios: Understanding the Differences
Table of Contents
When it comes to data fetching in React applications, two popular libraries often come up for debate: Axios vs React Query. Both offer solutions, but cater to different preferences.
Axios focuses on building clear, semantic models for managing your application state and data interactions. React Query, on the other hand, excels in simplifying data fetching tasks by handling common patterns like caching, refetching, and optimistic updates.
This blog explore the strengths of each approach to help you decide which one best suits your development style and project needs.
What are React Query and Axios?
Source - DhiWise
React Query is a library designed specifically for managing server state in React applications. It abstracts the complexities of data fetching by providing automatic data caching, refetching, and synchronization. This allows you to focus on building features without worrying about maintaining up-to-date data.
On the other hand, Axios is a promise-based HTTP client that simplifies sending HTTP requests and handling responses. It's known for its simplicity and wide browser support, making it a popular choice for developers who need to interact with RESTful APIs.
In the debate of "axios vs react query," it's important to understand how each tool fits into your development workflow and how they can complement each other.
Key Features of React Query
1. Automatic Caching and Synchronization
React Query automatically caches data, ensuring your application remains fast and efficient. The library handles stale data intelligently, updating the cache only when necessary. This approach reduces unnecessary network requests and keeps your UI in sync with the latest data.
2. Data Fetching and Mutations
React Query simplifies data-fetching operations with its powerful hooks, useQuery and useMutation. useQuery handles fetching and caching data, while useMutation manages data updates. This separation of concerns makes it easy to write clean and maintainable code.
- Polling: React Query supports polling, allowing you to refetch data at regular intervals automatically. This feature is useful for real-time applications that require continuous updates.
- Conditional Fetching: With React Query, you can conditionally fetch data based on specific criteria. This feature helps optimize network usage by only requesting data when needed.
3. Built-in Devtools
React Query comes with built-in devtools that enable developers to inspect and debug query states and cache. These tools provide insights into the behavior of your queries and help identify potential issues, making it easier to optimize your application's performance.
- Query Status: The devtools display the status of each query, such as loading, success, or error. This information is valuable for understanding the current state of your data fetching logic.
- Cache Inspection: The devtools allow you to inspect the cache, view cached data, and analyze cache updates. This capability helps you identify potential caching issues and optimize your cache configuration.
Example Code: Fetching Data with React Query
Here’s a quick example of how you might fetch data using React Query:
jsx
code
import { useQuery } from 'react-query';
import axios from 'axios';
const fetchData = async () => {
const { data } = await axios.get('https://api.example.com/data');
return data;
};
const MyComponent = () => {
const { data, error, isLoading } = useQuery('fetchData', fetchData);
if (isLoading) return Loading...;
if (error) return Error fetching data;
return Data: {JSON.stringify(data)};
};
Also read Best Tailwind Extension
Key Features of Axios
1. Promise-based HTTP Client
Axios simplifies making HTTP requests by using promises. This approach makes it easy to write clean and readable code without dealing with complex request configurations or callback hell. Axios also supports async/await syntax, further streamlining the code.
- Request Configuration: Axios provides a flexible configuration system, allowing you to set headers, query parameters, and other options globally or per request.
- Response Handling: Axios automatically transforms JSON data and provides a straightforward API for handling responses and errors.
2. Interceptors
Interceptors in Axios enable you to modify requests or responses before they are handled. This feature is useful for adding authentication tokens, logging request details, or handling errors globally.
Interceptor type | Description |
Request Interceptors | Modify outgoing requests by adding headers or altering request data. |
Response Interceptors | Handle responses consistently by transforming data or catching specific error codes. |
3. Wide Browser Support
Axios is compatible with older browsers, adhering to XMLHttpRequest standards. This makes it a reliable choice for projects that need to support various environments, including legacy browsers.
- Node.js Support: Axios is also compatible with Node.js, making it a versatile tool for both client-side and server-side applications.
- Cross-Domain Requests: Axios supports cross-domain requests and automatically handles CORS issues, simplifying API integrations.
import axios from 'axios';
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Axios vs React Query: Difference in use cases
React Query
It's ideal for managing server state. It excels in scenarios where automatic caching, refetching, and state synchronization are needed. React Query abstracts the complexities of state management, allowing developers to focus on building features.
- Optimistic Updates: React Query supports optimistic updates, allowing you to update the UI before a mutation is completed. This feature enhances user experience by providing instant feedback.
- Background Refetching: React Query can refetch data in the background when the app regains focus or when the user revisits a page. This ensures that users always see the most up-to-date data without interrupting their workflow.
Also read, React i18next Tutorial: Mastering Multilingual Support in Your React Application
Axios
It focuses on providing a simple and flexible way to make HTTP requests. It is best suited for applications where you need fine-grained control over request and response handling without additional overhead.
- Multipart Requests: Axios supports multipart requests, making it easy to upload files and handle form data.
- Custom Request Transformations: Axios allows you to customize request and response transformations, enabling advanced use cases like handling XML data or modifying request payloads.
Using React Query and Axios Together
Combining React Query and Axios lets you leverage the best of both worlds. Use Axios for making HTTP requests within React Query’s useQuery hook to take advantage of caching and synchronization. Here’s an example:
jsx
code
import { useQuery } from 'react-query';
import axios from 'axios';
const fetchDataWithAxios = async () => {
const { data } = await axios.get('https://api.example.com/data');
return data;
};
const MyComponent = () => {
const { data, error, isLoading } = useQuery('fetchData', fetchDataWithAxios);
if (isLoading) return Loading...;
if (error) return Error fetching data;
return Data: {JSON.stringify(data)};
};
This example demonstrates how you can combine the strengths of React Query and Axios to create a powerful data-fetching solution. By leveraging React Query's caching and state management capabilities with Axios's flexible HTTP client, you can build applications that are both efficient and easy to maintain.
Also read Website to React: CodeParrot Chrome Plugin
When to Use Axios vs React Query
- Use React Query when you need robust data synchronization, caching, and minimal manual state management. It’s perfect for applications that require real-time data updates and complex server state management.
- Use Axios when you need a straightforward way to make HTTP requests with fine control over request and response handling. It’s ideal for applications where simplicity and flexibility are paramount.
- In many cases, the best solution is to combine React Query and Axios. Use Axios to make HTTP requests and leverage React Query's powerful state management and caching capabilities. This approach allows you to build applications that are both efficient and maintainable.
Conclusion
In the debate of "axios vs react query," it's clear that both serve complementary roles in data fetching and management in React applications. Choosing between them depends on your project's specific requirements related to data fetching, caching, and synchronization.
React Query excels in managing server state, while Axios provides a simple and efficient way to handle HTTP requests. By combining both, you can create powerful, responsive applications that make the most of their respective strengths.
Looking to transform your website into a powerful React application? Discover how Code Parrot can help you seamlessly convert your existing site with our expert guidance and cutting-edge solutions. Don't miss the opportunity to enhance your web performance and user experience. Book a demo today and see the magic of React in action!
Related articles
Development using CodeParrot AI
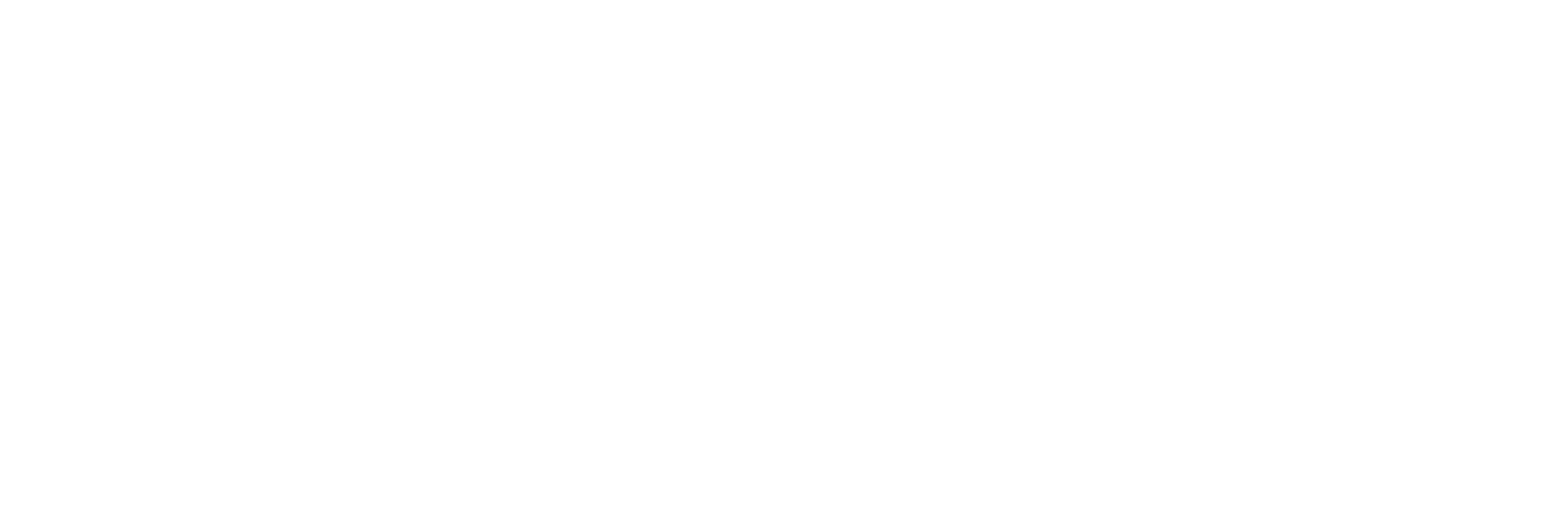