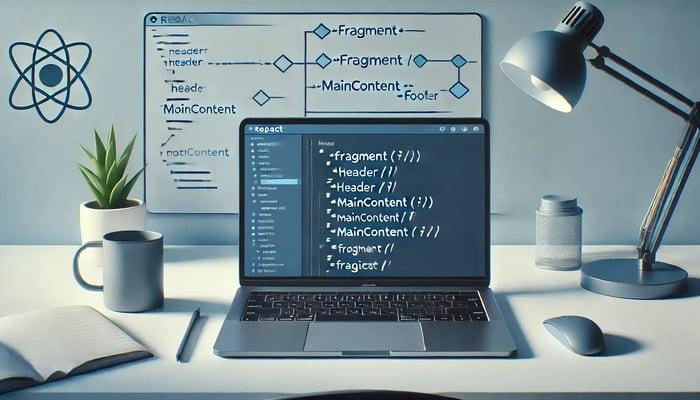
React Fragments: A Complete Guide
Meet Bob, a front-end developer at a startup. Bob is building a complex user interface using React, and he’s run into an issue that’s cluttering his DOM and causing layout problems. That’s when he discovers React Fragments, a feature that allows him to group a list of children without adding extra nodes to the DOM.
The Problem: Unnecessary <div>
Elements
Bob is working on a dashboard component that includes several child components: UserStats
, ActivityFeed
, and Notifications
. To render these components together, he wraps them in a <div>
:
function Dashboard() {
return (
<div>
<UserStats />
<ActivityFeed />
<Notifications />
</div>
);
}
However, Bob notices that this extra <div>
is causing issues with his CSS layout. The extra wrapper is interfering with his styling and causing alignment problems.
Issues Caused by Extra <div>
Elements
- Styling Conflicts: The additional
<div>
disrupts CSS selectors and layouts, making it harder to style components as intended. - Cluttered DOM: Unnecessary elements make the DOM tree more complex, which can make debugging more difficult.
- Performance Impact: Extra nodes can have a minor impact on rendering performance, especially in large applications.
The Solution: React Fragments
Bob remembers that React Fragments can help solve this problem by allowing him to return multiple elements without adding extra nodes to the DOM.
Implementing React Fragments
Bob updates his Dashboard
component to use Fragment
. You can also use <React.Fragment>
instead of <Fragment>
.
function Dashboard() {
return (
<Fragment>
<UserStats />
<ActivityFeed />
<Notifications />
</Fragment>
);
}
Using the Shorthand Syntax <> </>
Alternatively, Bob can use the shorthand syntax:
function Dashboard() {
return (
<>
<UserStats />
<ActivityFeed />
<Notifications />
</>
);
}
The Benefits
- Clean DOM Structure: No extra
<div>
elements are added to the DOM. - Simplified Styling: Without unnecessary wrappers, CSS selectors and layouts work as intended.
- Improved Readability: The code is cleaner and easier to understand.
Additional Features and Points About React Fragments
1. Using Keys with Fragments
When rendering a list of elements, you may need to assign a key
to each item. You can assign a key
to a fragment when using the full <Fragment>
syntax:
function ItemList({ items }) {
return (
<>
{items.map(item => (
<Fragment key={item.id}>
<ItemDetail item={item} />
</Fragment>
))}
</>
);
}
Note: The shorthand syntax <> </>
does not support attributes like key
.
2. Fragments in Tables
In HTML tables, you can’t wrap <td>
elements in a <div>
. React Fragments allow you to return multiple <td>
elements without adding extra nodes:
function TableRow({ data }) {
return (
<tr>
<Fragment>
<td>{data.firstName}</td>
<td>{data.lastName}</td>
<td>{data.email}</td>
</Fragment>
</tr>
);
}
3. Nesting Fragments
You can nest fragments inside other fragments or elements to organize your components:
function ComplexLayout() {
return (
<>
<Header />
<>
<MainContent />
<Sidebar />
</>
<Footer />
</>
);
}
4. Limitations of Fragments
- No Attributes on Shorthand Syntax: The shorthand
<> </>
cannot have attributes or props. - Cannot Use Refs: You cannot attach a
ref
to a fragment since it doesn’t correspond to a DOM node.
5. Improved Performance
By reducing the number of unnecessary DOM nodes, React Fragments can improve rendering performance, especially in large or complex applications.
Conclusion
By using React Fragments, Bob was able to clean up his code, simplify his DOM structure, and resolve styling issues caused by extra wrapper elements. React Fragments are a simple yet powerful tool that can help you write cleaner and more efficient React components.
Key Takeaways:
- Use React Fragments to Group Elements: Group child elements without adding extra nodes to the DOM.
- Use
<Fragment>
or<React.Fragment>
: You can use either<Fragment>
or<React.Fragment>
to create a fragment. - Use Full Syntax for Attributes: Use the full
<Fragment>
syntax when you need to add akey
or other attributes. - Use Shorthand Syntax for Simplicity: Use the shorthand
<> </>
syntax for simplicity when no attributes are needed. - Be Aware of Limitations: Remember that you cannot attach refs or attributes to the shorthand syntax.
References:
Related articles
Development using CodeParrot AI
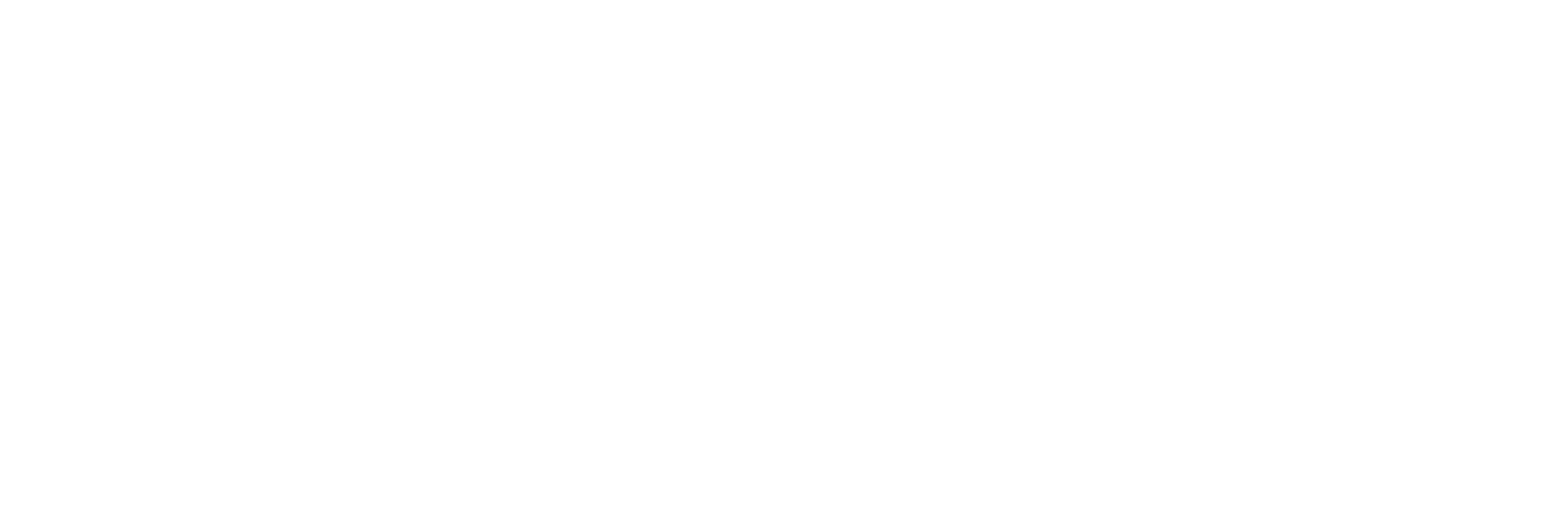