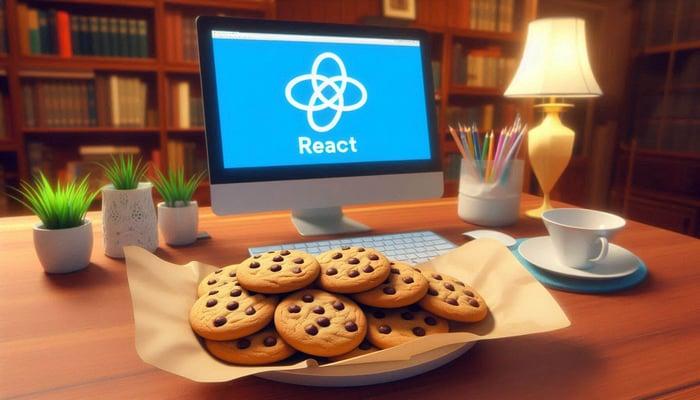
React Cookies: A Guide to Managing Cookies in React Apps
In the world of web development, managing state and user data is crucial for creating responsive, personalized experiences. One of the most common and versatile tools for this purpose is the humble cookie. But what exactly are cookies, and how can we harness their power in React applications? This blog post will dive deep into the world of React Cookies, exploring everything from basic concepts to advanced implementation techniques.
Cookies Basics
Cookies are small text files stored on the user's device. They serve as a memory for websites, allowing them to recognize users, remember preferences, and maintain state across page reloads or even separate visits.
These are the different types of cookies and their characteristics:
- Session Cookies: These temporary cookies last only as long as your browser session. They're deleted as soon as you close your browser window. Session cookies are perfect for storing short-term data like items in a shopping cart.
- Persistent Cookies: Unlike their sessional counterparts, persistent cookies have an expiration date. They remain on the user's device until that date, even if the browser is closed. These are ideal for remembering user preferences or login information.
- Secure Cookies: These cookies are only transmitted over HTTPS connections, adding an extra layer of security. They're crucial for handling sensitive information like authentication tokens.
- HttpOnly Cookies: These special cookies are inaccessible to JavaScript, making them resistant to cross-site scripting (XSS) attacks. They're often used for server-side session management.
Each type of cookie has its use cases and understanding them is key to implementing effective and secure cookie strategies in your React applications.
Why and When to Use Cookies in React
Cookies are an integral part of web development, but it's important to understand why and when to use them in your React application. While there are several alternatives like localStorage
and sessionStorage
, cookies offer unique advantages that make them suitable for specific use cases.
Why Use Cookies?
• Persistent Storage Across Sessions: Cookies are ideal for storing data that needs to persist across multiple sessions. Unlike localStorage
and sessionStorage
, which are client-side only, cookies can be sent to the server with every HTTP request, allowing for seamless persistence and data retrieval.
• Secure Data Transmission: When security is a concern, cookies are often preferred over other storage mechanisms. By using the secure
and httpOnly
flags, you can ensure that sensitive information like authentication tokens is transmitted over secure channels and protected from client-side access, reducing the risk of XSS attacks.
• Server-Side Interaction: Cookies are automatically included in HTTP requests sent to the server. This makes them particularly useful for server-side tasks like session management, user authentication, and tracking user behavior.
When to Use Cookies?
• User Authentication: Cookies are often used to store session tokens or JWTs that authenticate users across different parts of an application. This allows users to remain logged in as they navigate through the app, and the server can verify their identity with each request.
• Storing User Preferences: Cookies can store user preferences such as theme settings, language choices, and other personalized configurations. This ensures that users have a consistent experience every time they visit your application, even across different devices.
• Tracking User Behavior: Cookies can be used to track user behavior across sessions, helping you gather insights into how users interact with your application. This data can be valuable for analytics, personalization, and improving the user experience.
When Not to Use Cookies?
While cookies are powerful, they are not always the best choice. Here are a few scenarios where alternatives might be better:
• Storing Large Data: Cookies are limited in size (typically 4KB), so they are not suitable for storing large amounts of data. For larger datasets, consider using localStorage
, sessionStorage
, or IndexedDB
.
• Client-Side Only Data: If the data does not need to be sent to the server with every request, localStorage
or sessionStorage
might be more appropriate. These options provide more storage capacity and do not add to the size of HTTP requests.
• Temporary Data: For data that only needs to persist for the duration of a session and does not require server-side validation, sessionStorage
is a simpler alternative. It automatically clears when the browser session ends.
Working with Cookies in React
When it comes to handling cookies in React, you have two main options: using native JavaScript methods or leveraging third-party libraries. While the native approach gives you fine-grained control, libraries can significantly simplify cookie management.
Let's set up a project using a popular cookie library, js-cookie
:
npm install js-cookie
This library provides a clean, easy-to-use API for cookie manipulation. Here's how you can import it in your React component:
import Cookies from 'js-cookie';
// Set a cookie
Cookies.set('name', 'value', { expires: 7 });
// Get a cookie
const value = Cookies.get('name');
// Remove a cookie
Cookies.remove('name');
This code demonstrates the basic operations with js-cookie
:
Cookies.set()
: This function creates a new cookie. It takes three arguments: the cookie name, its value, and an options object. Here, we're setting a cookie namedname
with the valuevalue
that will expire in 7 days.Cookies.get()
: This function retrieves the value of a cookie. In this case, it's fetching the value of thename
cookie we just set.Cookies.remove()
: This function deletes a cookie. It's removing thename
cookie from the browser.
These operations form the foundation of cookie management in React applications.
Creating and Setting React Cookies
Setting cookies is often the first step in implementing cookie-based features. Let's explore how to create cookies with various options:
import Cookies from 'js-cookie';
// Set a simple cookie
Cookies.set('username', 'JohnDoe');
// Set a cookie that expires in 7 days
Cookies.set('rememberMe', 'true', { expires: 7 });
// Set a secure cookie (only transmitted over HTTPS)
Cookies.set('authToken', 'abc123', { secure: true });
// Set a cookie with path and domain
Cookies.set('preference', 'darkMode', { path: '/dashboard', domain: 'example.com' });
In these examples, we're setting cookies with different options:
- A basic cookie with just a name and value. This is the simplest form of cookie, useful for storing temporary data.
- A cookie with an expiration date. The
expires
option sets the cookie to last for 7 days, after which it will be automatically deleted by the browser. This is useful for features like "Remember me" on login forms. - A secure cookie for sensitive data. The
secure: true
option ensures that this cookie is only transmitted over HTTPS connections, adding an extra layer of security. This is crucial for storing sensitive information like authentication tokens. - A cookie with specific path and domain restrictions. The
path
option restricts the cookie to only be accessible on the specified path ('/dashboard' in this case), while thedomain
option sets the domain for which the cookie is valid. This allows for more granular control over where and when the cookie can be accessed.
Reading and Accessing Cookies
Once you've set cookies, you'll need to read them to make use of the stored data. Here's how you can access cookie values:
import Cookies from 'js-cookie';
// Read a cookie value
const username = Cookies.get('username');
console.log(username); // 'JohnDoe'
// Check if a cookie exists
if (Cookies.get('authToken')) {
console.log('User is authenticated');
} else {
console.log('User is not authenticated');
}
// Get all cookies
const allCookies = Cookies.get();
console.log(allCookies); // { username: 'JohnDoe', rememberMe: 'true', ... }
This code demonstrates different ways to read and access cookies:
Cookies.get('username')
: This retrieves the value of the 'username' cookie. It's a simple way to access a specific cookie's value.- The
if
statement checks if the 'authToken' cookie exists. This is a common pattern for checking if a user is authenticated. If the cookie exists, it assumes the user is authenticated. Cookies.get()
without arguments returns an object containing all cookies. This is useful when you need to access multiple cookies at once.
When working with cookies, always handle the case where a cookie might not exist. Here's a more robust example:
function getUserPreference(key, defaultValue) {
const value = Cookies.get(key);
return value !== undefined ? value : defaultValue;
}
const theme = getUserPreference('theme', 'light');
console.log(`Using ${theme} theme`); // 'Using light theme' if cookie is not set
This approach provides a fallback value, ensuring your app behaves predictably even if the expected cookie is missing.
Updating and Deleting React Cookies
As your React application's state changes, you may need to update or remove cookies. Here's how to manage these operations:
import Cookies from 'js-cookie';
// Update a React cookie
Cookies.set('username', 'JaneDoe'); // Overwrites the existing 'username' cookie
// Remove a specific React cookie
Cookies.remove('rememberMe');
// Clear all React cookies
Object.keys(Cookies.get()).forEach(cookie => {
Cookies.remove(cookie);
});
This code demonstrates how to update and delete cookies:
- Updating a cookie is done by simply setting it again with the same name. This overwrites the existing cookie with the new value.
Cookies.remove('rememberMe')
deletes the specific 'rememberMe' cookie. This is useful when you no longer need to store that particular piece of information.- The last part shows how to clear all cookies. It first gets all cookie names using
Object.keys(Cookies.get())
, then iterates over them, removing each cookie. This can be useful for operations like logging out a user, where you want to clear all stored data.
React Authentication Cookies
Cookies are frequently used for maintaining user sessions in React apps. Here's an example of how you might implement cookie-based authentication in React:
import Cookies from 'js-cookie';
function login(username, password) {
return api.login(username, password).then(response => {
if (response.success) {
Cookies.set('authToken', response.token, {
expires: 7,
secure: true,
sameSite: 'strict'
});
return true;
}
return false;
});
}
function logout() {
Cookies.remove('authToken');
}
function checkAuth() {
return Cookies.get('authToken') != null;
}
// Usage in a React component
function LoginButton() {
const [isLoggedIn, setIsLoggedIn] = useState(checkAuth());
const handleLogin = async () => {
const success = await login('user', 'pass');
setIsLoggedIn(success);
};
const handleLogout = () => {
logout();
setIsLoggedIn(false);
};
return isLoggedIn ? (
<button onClick={handleLogout}>Logout</button>
) : (
<button onClick={handleLogin}>Login</button>
);
}
This code demonstrates a basic implementation of cookie-based authentication:
- The
login
function calls an API to authenticate the user. If successful, it sets anauthToken
cookie with the received token. The cookie is set to expire in 7 days, is marked assecure
(HTTPS only), and uses a strict same-site policy for added security. - The
logout
function simply removes theauthToken
cookie. checkAuth
checks if theauthToken
cookie exists, returning true if it does (indicating the user is logged in).- The
LoginButton
component uses React'suseState
hook to keep track of the login state. It provides methods for handling login and logout actions. It also renders either aLogin
orLogout
button based on the current authentication state.
Best Practices for Managing Cookies
When working with cookies in React, following best practices is essential to ensure security, performance, and user experience.
1. Use Secure and HttpOnly Flags: Always use the secure
and httpOnly
flags for cookies that contain sensitive information, such as authentication tokens. This prevents exposure to XSS attacks and ensures secure transmission.
2. Set an Expiration Date: Define an expiration date for your cookies to prevent them from persisting indefinitely. This is especially important for session cookies, where you want to control how long a user remains logged in.
5. Handle Cross-Site Scripting (XSS): Protect your application from XSS attacks by ensuring that cookies cannot be accessed or modified by malicious scripts. This can be achieved using the httpOnly
flag and sanitizing any user input that interacts with cookies.
For more information, refer to the MDN Web Docs on Cookies.
Conclusion
React cookies are a powerful tool for managing state and user data in React applications. From basic storage to complex authentication systems, mastering React cookie management opens up a world of possibilities for creating dynamic, personalized user experiences.
Remember, while being beneficial, they should be used judiciously. Always consider the privacy and security implications of storing data in cookies and explore alternative state management solutions when appropriate. With this knowledge, you're now ready to leverage the full power of React cookies in your applications!
Related articles
Development using CodeParrot AI
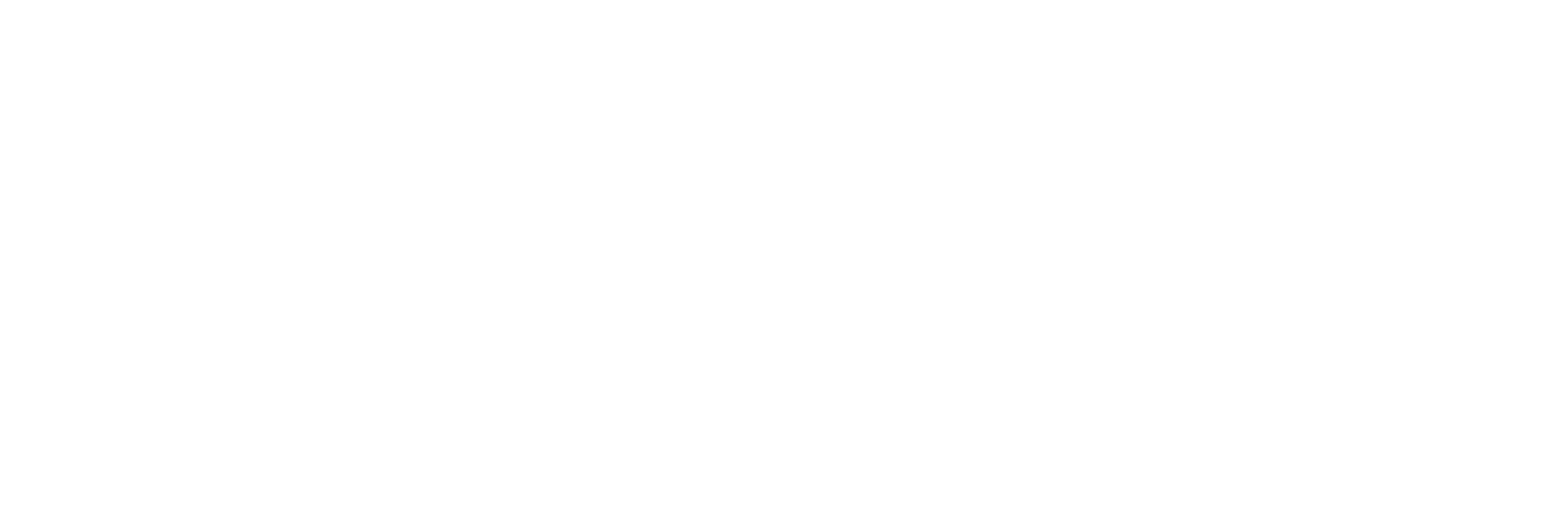